[Q#10039672][A#10039728] Android : How to read file in bytes?
https://stackoverflow.com/q/10039672
I am trying to get file content in bytes in Android application. I have get the file in SD card now want to get the selected file in bytes. I googled but no such success. Please help Below is the code to get files with extension. Through this i get files and show in spinner. On file selection I want to get file in bytes.
Answer
https://stackoverflow.com/a/10039728
here it's a simple: Add permission in manifest.xml:
APIzation

File file = new File(path);
int size = (int) file.length();
byte[] bytes = new byte[size];
try {
BufferedInputStream buf = new BufferedInputStream(new FileInputStream(file));
buf.read(bytes, 0, bytes.length);
buf.close();
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
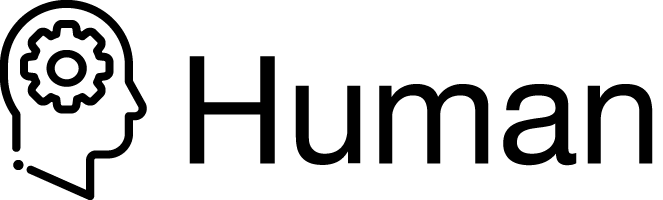
package com.stackoverflow.api;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class Human10039728 {
public static byte[] readFileInByte(String path) throws IOException {
File file = new File(path);
int size = (int) file.length();
byte[] bytes = new byte[size];
BufferedInputStream buf = new BufferedInputStream(
new FileInputStream(file)
);
buf.read(bytes, 0, bytes.length);
buf.close();
return bytes;
}
}
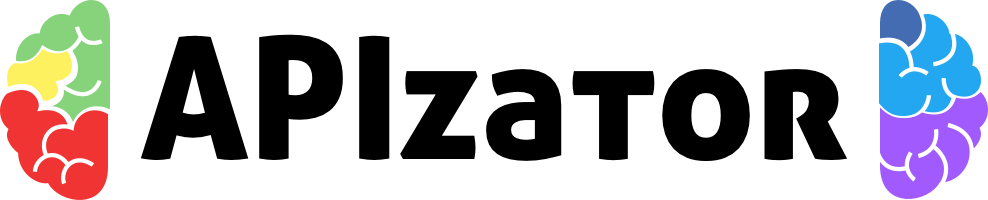
package com.stackoverflow.api;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/**
* Android : How to read file in bytes?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/10039728">https://stackoverflow.com/a/10039728</a>
*/
public class APIzator10039728 {
public static void android(String path) throws Exception {
File file = new File(path);
int size = (int) file.length();
byte[] bytes = new byte[size];
try {
BufferedInputStream buf = new BufferedInputStream(
new FileInputStream(file)
);
buf.read(bytes, 0, bytes.length);
buf.close();
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}