[Q#1073919][A#1073933] How to convert int[] into List<Integer> in Java?
https://stackoverflow.com/q/1073919
How do I convert int[] into List<Integer> in Java? Of course, I'm interested in any other answer than doing it in a loop, item by item. But if there's no other answer, I'll pick that one as the best to show the fact that this functionality is not part of Java.
Answer
https://stackoverflow.com/a/1073933
There is no shortcut for converting from int[] to List<Integer> as Arrays.asList does not deal with boxing and will just create a List<int[]> which is not what you want. You have to make a utility method.
APIzation

int[] ints = {1, 2, 3};
List<Integer> intList = new ArrayList<Integer>();
for (int i : ints)
{
intList.add(i);
}
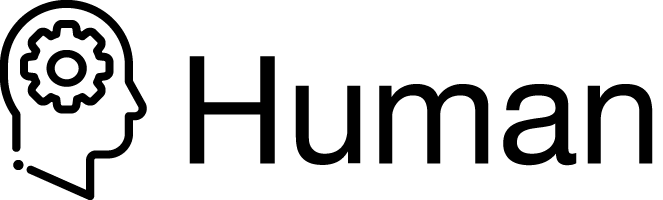
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
public class Human1073933 {
public static List<Integer> toList(int[] ints) {
List<Integer> intList = new ArrayList<>();
for (int i : ints) {
intList.add(i);
}
return intList;
}
}
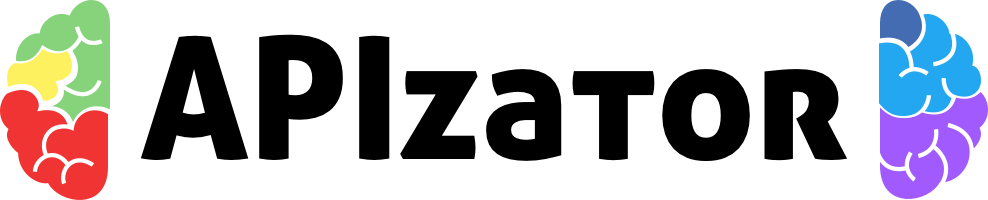
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
/**
* How to convert int[] into List<Integer> in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/1073933">https://stackoverflow.com/a/1073933</a>
*/
public class APIzator1073933 {
public static void convertInt(int[] ints) throws Exception {
List<Integer> intList = new ArrayList<Integer>();
for (int i : ints) {
intList.add(i);
}
}
}