[Q#12812307][A#12812355] How to remove specific value from string array in java?
https://stackoverflow.com/q/12812307
Possible Duplicate: Removing an element from an Array (Java) How to remove specific String array value for example String[] str_array = {"item1","item2","item3"}; i want to remove "item2" from str_array pls help me i want output like String[] str_array = {"item1","item3"};
Answer
https://stackoverflow.com/a/12812355
I would do it as follows:
APIzation

String[] str_array = {"item1","item2","item3"};
List<String> list = new ArrayList<String>(Arrays.asList(str_array));
list.remove("item2");
str_array = list.toArray(new String[0]);
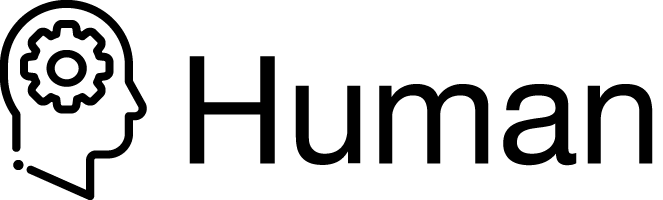
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Human12812355 {
public static String[] removeItemFromStringArray(
String[] str_array,
String item
) {
List<String> list = new ArrayList<String>(Arrays.asList(str_array));
list.remove(item);
str_array = list.toArray(new String[0]);
return str_array;
}
}
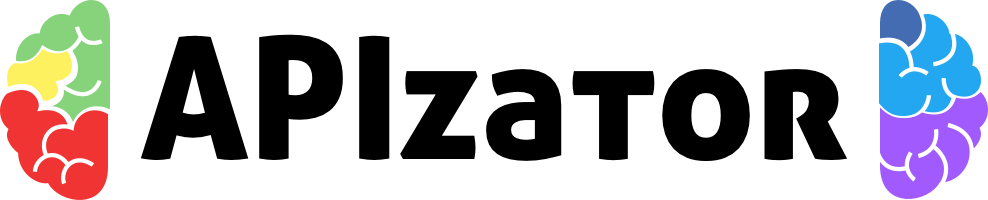
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* How to remove specific value from string array in java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/12812355">https://stackoverflow.com/a/12812355</a>
*/
public class APIzator12812355 {
public static void removeValue(String[] str_array) throws Exception {
List<String> list = new ArrayList<String>(Arrays.asList(str_array));
list.remove("item2");
str_array = list.toArray(new String[0]);
}
}