[Q#12899848][A#12899896] How to add one month to a date and get the same day
https://stackoverflow.com/q/12899848
I try to add one month to a date depending on weekdays. Fore example the date is the 3. Monday of September. After adding I want have the 3. Monday of October. I tried to add one month to following date with this code: But I got which is the second Monday of Oct and not the third. Has anybody an idea how this workes? EDIT This is the solution I used like in the answer below: wereby week is the number of the week in a month. Fore example 1 means the first, 2 the second, and so on. But week can also count backwards, fore example -1 means the last week of month.
Answer
https://stackoverflow.com/a/12899896
If you want get 3rd monday of month, then use set instead of add date.set(Calendar.DAY_OF_WEEK_IN_MONTH, 3); if you want add one month to current date, use date.add(Calendar.MONTH, 1); EDIT
APIzation

final Calendar date = Calendar.getInstance();
date.set(2012, Calendar.SEPTEMBER, 17);
int prevDayOfWeekInMonth = date.get(Calendar.DAY_OF_WEEK_IN_MONTH);
int prevDayOfWeek = date.get(Calendar.DAY_OF_WEEK);
date.add(Calendar.MONTH, 1);
date.set(Calendar.DAY_OF_WEEK, prevDayOfWeek);
date.set(Calendar.DAY_OF_WEEK_IN_MONTH, prevDayOfWeekInMonth);
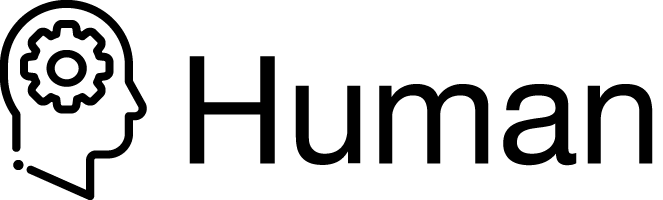
package com.stackoverflow.api;
import java.util.Calendar;
public class Human12899896 {
public static Calendar getTheSameDayInAMonth(int year, int month, int day) {
final Calendar date = Calendar.getInstance();
date.set(year, month, day);
int prevDayOfWeekInMonth = date.get(Calendar.DAY_OF_WEEK_IN_MONTH);
int prevDayOfWeek = date.get(Calendar.DAY_OF_WEEK);
date.add(Calendar.MONTH, 1);
date.set(Calendar.DAY_OF_WEEK, prevDayOfWeek);
date.set(Calendar.DAY_OF_WEEK_IN_MONTH, prevDayOfWeekInMonth);
return date;
}
}
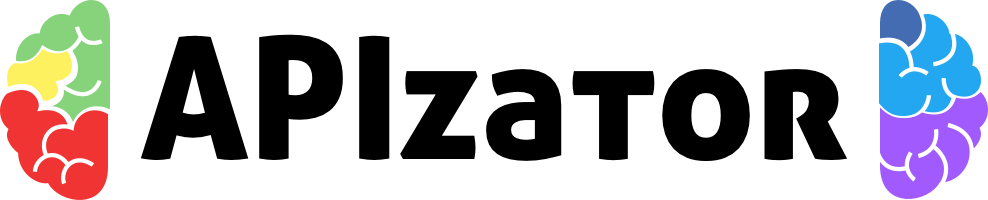
package com.stackoverflow.api;
import java.util.Calendar;
/**
* How to add one month to a date and get the same day
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/12899896">https://stackoverflow.com/a/12899896</a>
*/
public class APIzator12899896 {
public static void addMonth() throws Exception {
final Calendar date = Calendar.getInstance();
date.set(2012, Calendar.SEPTEMBER, 17);
int prevDayOfWeekInMonth = date.get(Calendar.DAY_OF_WEEK_IN_MONTH);
int prevDayOfWeek = date.get(Calendar.DAY_OF_WEEK);
date.add(Calendar.MONTH, 1);
date.set(Calendar.DAY_OF_WEEK, prevDayOfWeek);
date.set(Calendar.DAY_OF_WEEK_IN_MONTH, prevDayOfWeekInMonth);
}
}