[Q#13021102][A#13021152] In java, how would I find the nth Fibonacci number?
https://stackoverflow.com/q/13021102
Determining the Fibonacci sequence is easy enough to figure out: My problem lies with trying to pinpoint the value for a specified N. As in, If I want to find the 6th element in the sequence, which is 8, how would I find that number, and only that number?
Answer
https://stackoverflow.com/a/13021152
In your code, num starts as the 0th Fibonacci number, and num1 as the 1st. So to find the nth, you have to iterate the step n times: and only print it when you've finished. When the loop counter loop has the value k, num holds the kth Fibonacci number and num2 the (k+1)th.
APIzation

for (loop = 0; loop < n; loop ++)
{
fibonacci = num + num2;
num = num2;
num2 = fibonacci;
}
System.out.print(num);
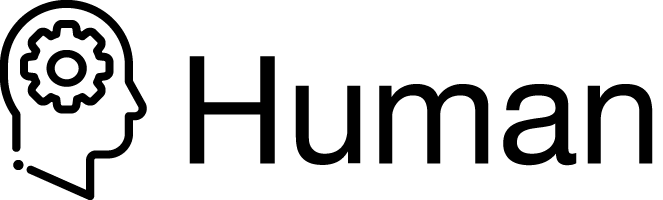
package com.stackoverflow.api;
public class Human13021152 {
public static int findNthFibonacciNumber(int n) {
int num = 0;
int num2 = 1;
int loop;
int fibonacci;
System.out.print(num2);
for (loop = 0; loop < n; loop++) {
fibonacci = num + num2;
num = num2;
num2 = fibonacci;
}
System.out.print(num);
return num;
}
}
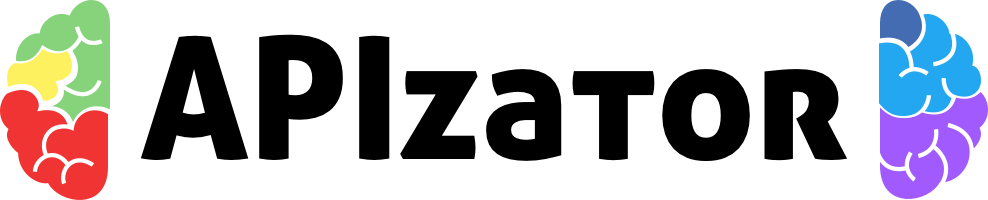
package com.stackoverflow.api;
/**
* In java, how would I find the nth Fibonacci number?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/13021152">https://stackoverflow.com/a/13021152</a>
*/
public class APIzator13021152 {
public static void findNumber(int n) throws Exception {
int num2 = 0;
int num = 0;
int loop = 0;
int fibonacci = 0;
for (loop = 0; loop < n; loop++) {
fibonacci = num + num2;
num = num2;
num2 = fibonacci;
}
System.out.print(num);
}
}