[Q#1509391][A#1509487] how to get the one entry from hashmap without iterating
https://stackoverflow.com/q/1509391
Is there a elegant way of obtaining only one Entry<K,V> from HashMap, without iterating, if key is not known. As order of entry of entry is not important, can we say something like Although I am aware that there exist no such get by index method. If I tried approach mentioned below, it would still have to get all the entry set of the hashmap. Suggestions are welcome. EDIT: Please suggest any other Data Structure to suffice requirement.
Answer
https://stackoverflow.com/a/1509487
The answer by Jesper is good. An other solution is to use TreeMap (you asked for other data structures). TreeMap has an overhead so HashMap is faster, but just as an example of an alternative solution.
APIzation

TreeMap<String, String> myMap = new TreeMap<String, String>();
String first = myMap.firstEntry().getValue();
String firstOther = myMap.get(myMap.firstKey());
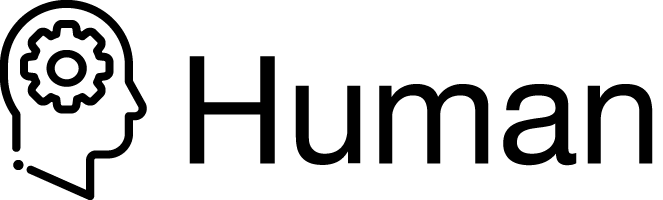
package com.stackoverflow.api;
import java.util.TreeMap;
public class Human1509487 {
public static String methodName(TreeMap<String, String> myMap) {
String first = myMap.firstEntry().getValue();
return myMap.get(myMap.firstKey());
}
}
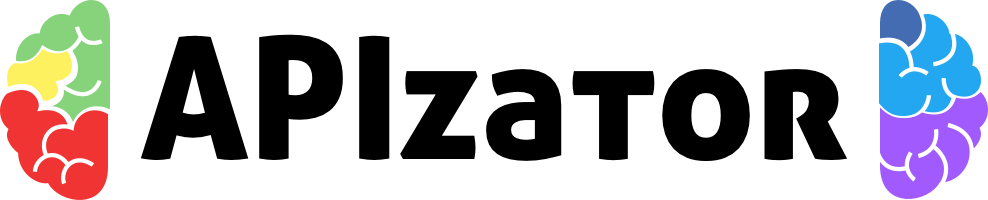
package com.stackoverflow.api;
import java.util.TreeMap;
/**
* how to get the one entry from hashmap without iterating
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/1509487">https://stackoverflow.com/a/1509487</a>
*/
public class APIzator1509487 {
public static String getEntry() throws Exception {
TreeMap<String, String> myMap = new TreeMap<String, String>();
String first = myMap.firstEntry().getValue();
return myMap.get(myMap.firstKey());
}
}