[Q#15150193][A#15150223] How to check that a string contains characters other than those specified. (in Java)
https://stackoverflow.com/q/15150193
I have a program that asks the user to input a three character string. The string can only be a combination of a, b, or c. How do I check if the string contains any other characters than those specified without doing a million conditional statements. Pseudo example:
Answer
https://stackoverflow.com/a/15150223
To look for characters that are NOT a, b, or c, use something like the following:
APIzation

if(!s.matches("[abc]+"))
{
System.out.println("The string you entered has some incorrect characters");
}
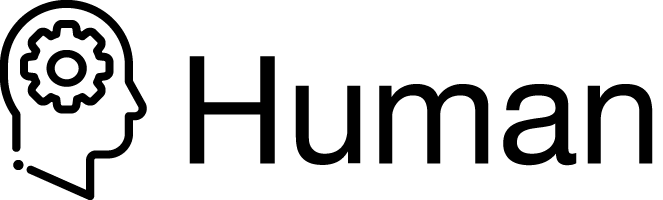
package com.stackoverflow.api;
public class Human15150223 {
public static boolean stringMatchesCharactersExclusively(
String s,
String characters
) {
//https://stackoverflow.com/a/15150223
if (!s.matches("[" + characters + "]+")) {
System.out.println(
"The string you entered has some incorrect characters"
);
return false;
}
return true;
}
}
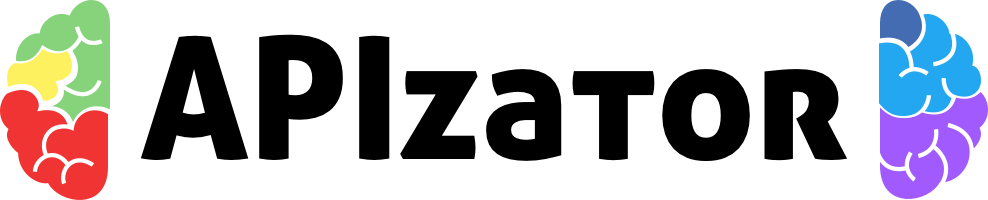
package com.stackoverflow.api;
/**
* How to check that a string contains characters other than those specified. (in Java)
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/15150223">https://stackoverflow.com/a/15150223</a>
*/
public class APIzator15150223 {
public static void check(String s) throws Exception {
if (!s.matches("[abc]+")) {
System.out.println(
"The string you entered has some incorrect characters"
);
}
}
}