[Q#15518845][A#15518922] How to validate URL in java using regex?
https://stackoverflow.com/q/15518845
I want to validate url started with http/https/www/ftp and checks for /\ slashes and checks for .com,.org etc at the end of URL using regular expression. Is there any regex patttern for URL validation?
Answer
https://stackoverflow.com/a/15518922
This works:
APIzation

Pattern p = Pattern.compile("(@)?(href=')?(HREF=')?(HREF=\")?(href=\")?(http://)?[a-zA-Z_0-9\\-]+(\\.\\w[a-zA-Z_0-9\\-]+)+(/[#&\\n\\-=?\\+\\%/\\.\\w]+)?");
Matcher m = p.matcher("your url here");
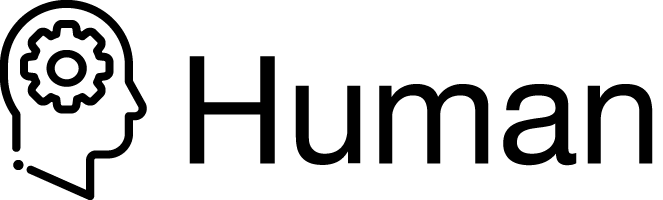
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human15518922 {
public static boolean validateURLInJavaUsingRegex(String url) {
Pattern p = Pattern.compile(
"(@)?(href=')?(HREF=')?(HREF=\")?(href=\")?(http://)?[a-zA-Z_0-9\\-]+(\\.\\w[a-zA-Z_0-9\\-]+)+(/[#&\\n\\-=?\\+\\%/\\.\\w]+)?"
);
Matcher m = p.matcher(url);
return m.matches();
}
}
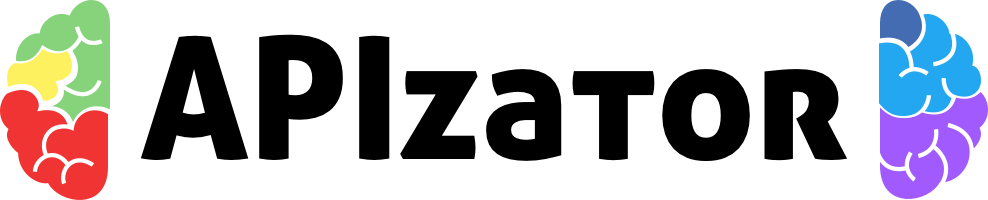
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How to validate URL in java using regex?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/15518922">https://stackoverflow.com/a/15518922</a>
*/
public class APIzator15518922 {
public static Matcher validateUrl() throws Exception {
Pattern p = Pattern.compile(
"(@)?(href=')?(HREF=')?(HREF=\")?(href=\")?(http://)?[a-zA-Z_0-9\\-]+(\\.\\w[a-zA-Z_0-9\\-]+)+(/[#&\\n\\-=?\\+\\%/\\.\\w]+)?"
);
return p.matcher("your url here");
}
}