[Q#15977137][A#15977262] How to append a byte to a string in Java?
https://stackoverflow.com/q/15977137
I have this operation I need to perform where I need to append a byte such as 0x10 to some String in Java. I was wondering how I could go about doing this? For example: In this example, how would I go about appending someByte to someString? The reason why I am asking this question is because the application I am developing is supposed to send commands to some server. The server is able to accept commands (base64 encoded), decode the command, and parse out these bytes that are not necessarily compatible with any sort of ASCII encoding standard for performing some special function.
Answer
https://stackoverflow.com/a/15977262
If you want to concatenate the actual value of a byte to a String use the Byte wrapper and its toString() method, like this:
APIzation

String someString = "STRING";
byte someByte = 0x10;
someString += Byte.toString(someByte);
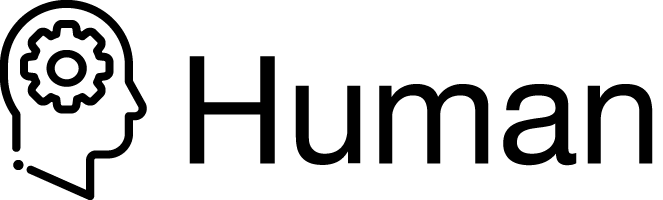
package com.stackoverflow.api;
public class Human15977262 {
public static String byteToString(byte someByte, String someString) {
return someString + Byte.toString(someByte);
}
}
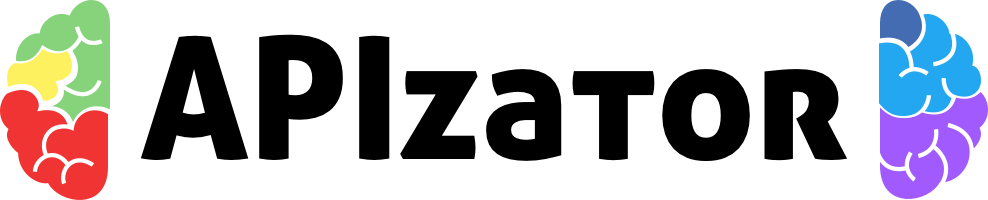
package com.stackoverflow.api;
/**
* How to append a byte to a string in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/15977262">https://stackoverflow.com/a/15977262</a>
*/
public class APIzator15977262 {
public static void appendByte(String someString, byte someByte)
throws Exception {
someString += Byte.toString(someByte);
}
}