[Q#16247623][A#16247861] How to read from a file and save the content into a linked list?
https://stackoverflow.com/q/16247623
I'm trying to write the code for a program that saves the content of a txt file into a singly linked list, every line in the txt file represent an object. I used Scanner to read from the file but I don't know how to get the words in the list. any tip or hint is highly appreciated. my attempt: class Item class Node class ListItems class FileItems
Answer
https://stackoverflow.com/a/16247861
Open the text file and read each line as a String and place that String object into a LinkedList. Print all of the lines in the LinkedList in reverse order.
APIzation

import java.util.*;
import java.io.*;
public class FileLinkList
{
public static void main(String args[])throws IOException{
String content = new String();
int count=1;
File file = new File("abc.txt");
LinkedList<String> list = new LinkedList<String>();
try {
Scanner sc = new Scanner(new FileInputStream(file));
while (sc.hasNextLine()){
content = sc.nextLine();
list.add(content);
}
sc.close();
}catch(FileNotFoundException fnf){
fnf.printStackTrace();
}
catch (Exception e) {
e.printStackTrace();
System.out.println("\nProgram terminated Safely...");
}
Collections.reverse(list);
Iterator i = list.iterator();
while (i.hasNext()) {
System.out.print("Node " + (count++) + " : ");
System.out.println(i.next());
}
}
}
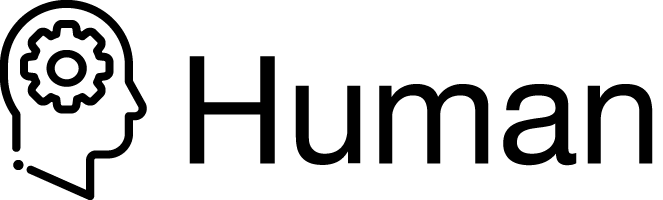
package com.stackoverflow.api;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.util.Collections;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Scanner;
public class Human16247861 {
public static LinkedList readFileIntoLinkedList(File file) {
String content = new String();
int count = 1;
LinkedList<String> list = new LinkedList<>();
try {
Scanner sc = new Scanner(new FileInputStream(file));
while (sc.hasNextLine()) {
content = sc.nextLine();
list.add(content);
}
sc.close();
} catch (FileNotFoundException fnf) {
fnf.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
System.out.println("\nProgram terminated Safely...");
}
Collections.reverse(list);
Iterator i = list.iterator();
while (i.hasNext()) {
System.out.print("Node " + (count++) + " : ");
System.out.println(i.next());
}
return list;
}
}
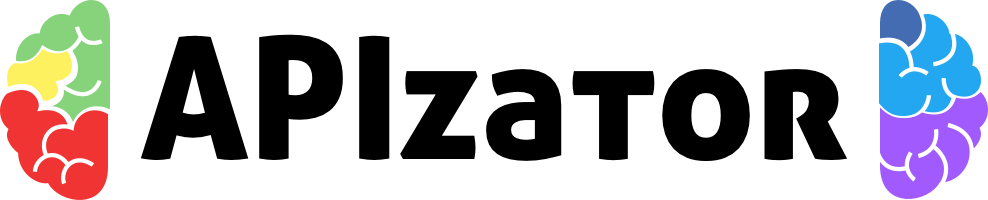
package com.stackoverflow.api;
import java.io.*;
import java.util.*;
/**
* How to read from a file and save the content into a linked list?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/16247861">https://stackoverflow.com/a/16247861</a>
*/
public class APIzator16247861 {
public static void read(String str1) throws IOException {
String content = new String();
int count = 1;
File file = new File(str1);
LinkedList<String> list = new LinkedList<String>();
try {
Scanner sc = new Scanner(new FileInputStream(file));
while (sc.hasNextLine()) {
content = sc.nextLine();
list.add(content);
}
sc.close();
} catch (FileNotFoundException fnf) {
fnf.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
System.out.println("\nProgram terminated Safely...");
}
Collections.reverse(list);
Iterator i = list.iterator();
while (i.hasNext()) {
System.out.print("Node " + (count++) + " : ");
System.out.println(i.next());
}
}
}