[Q#16360720][A#16361505] How to find out number of files currently open by Java application?
https://stackoverflow.com/q/16360720
Suppose a lot of what your application does deals with reading contents of the files. Goes without saying that files that are open then closed and life is good unless .. new files come in faster then old files get closed. This is the pickle of a situation i found myself in. Now .. is there a way to reliably know how many files are open by the process? Something that as reliable as looking at ls /proc/my_pid/fd | wc -l from inside the JVM? I suspect answer may be OS specific, so let me add that i am running Java on Linux.
Answer
https://stackoverflow.com/a/16361505
On unix one way is using the ManagementFactory to get the OperatingSystemMxBean and if it is a UnixOperatingSystemMXBean you can use getOpenFileDescriptorCount() method. Example Code below
APIzation

import java.lang.management.ManagementFactory;
import java.lang.management.OperatingSystemMXBean;
import com.sun.management.UnixOperatingSystemMXBean;
public class OpenFileCount{
public static void main(String[] args){
OperatingSystemMXBean os = ManagementFactory.getOperatingSystemMXBean();
if(os instanceof UnixOperatingSystemMXBean){
System.out.println("Number of open fd: " + ((UnixOperatingSystemMXBean) os).getOpenFileDescriptorCount());
}
}
}
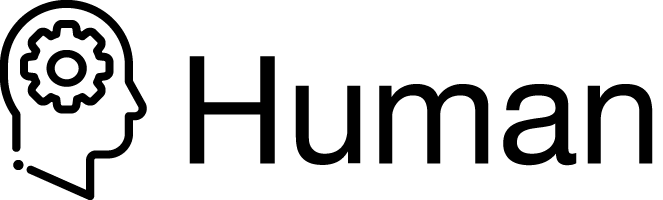
package com.stackoverflow.api;
import com.sun.management.UnixOperatingSystemMXBean;
import java.lang.management.ManagementFactory;
import java.lang.management.OperatingSystemMXBean;
public class Human16361505 {
public static int numberOfOpenFileDescriptor() {
OperatingSystemMXBean os = ManagementFactory.getOperatingSystemMXBean();
if (os instanceof UnixOperatingSystemMXBean) {
return ((UnixOperatingSystemMXBean) os).getOpenFileDescriptorCount();
}
return -1;
}
}
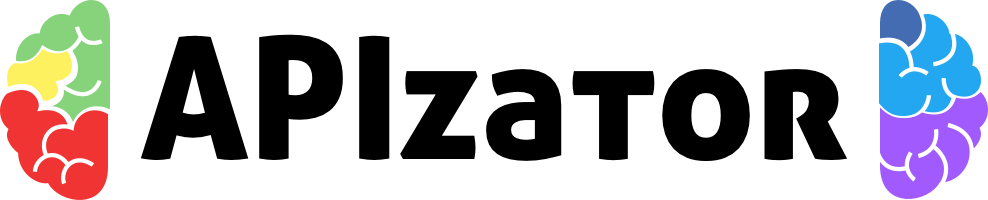
package com.stackoverflow.api;
import com.sun.management.UnixOperatingSystemMXBean;
import java.lang.management.ManagementFactory;
import java.lang.management.OperatingSystemMXBean;
/**
* How to find out number of files currently open by Java application?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/16361505">https://stackoverflow.com/a/16361505</a>
*/
public class APIzator16361505 {
public static void find() {
OperatingSystemMXBean os = ManagementFactory.getOperatingSystemMXBean();
if (os instanceof UnixOperatingSystemMXBean) {
System.out.println(
"Number of open fd: " +
((UnixOperatingSystemMXBean) os).getOpenFileDescriptorCount()
);
}
}
}