[Q#18070629][A#18070669] How to read from user's input in Java and write it to a file
https://stackoverflow.com/q/18070629
I want to create a simple stand-alone application that will take some input from user (some numbers and mathematical functions f(x,y…)) and write them to a file. Then with the help of this file I will run a command. Basic ingredients that I need: – JTextArea for users input. – ButtonHandler/ActionListener and writing of the input to a (txt) file – ButtonHandler/ActionLister to execute a command What is the best way to do it? A current running code that I have (basically a toy) - which does not write anything, just executes - is: In the above example how can I write 'value' to a file? Then, how can I add more input (more textfields)? Can I do it in the same class or I need more? My confusion comes (mainly but not only) from the fact that inside the ButtonHandler class I can NOT define any other objects (ie, open and write files etc).
Answer
https://stackoverflow.com/a/18070669
This is the way I would write to a file. I will let you convert this code into your GUI for practice. See more on BufferedWriter and FileWriter
APIzation

import java.io.BufferedWriter;
import java.io.FileWriter;
import java.util.Scanner;
public class Files {
public static void main(String args[]){
System.out.print("Enter Text: ");
Scanner scan = new Scanner(System.in);
String text = scan.nextLine();
FileWriter fWriter = null;
BufferedWriter writer = null;
try {
fWriter = new FileWriter("text.txt");
writer = new BufferedWriter(fWriter);
writer.write(text);
writer.newLine();
writer.close();
System.err.println("Your input of " + text.length() + " characters was saved.");
} catch (Exception e) {
System.out.println("Error!");
}
}
}
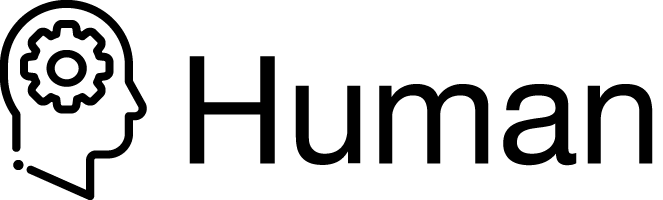
package com.stackoverflow.api;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.util.Scanner;
public class Human18070669 {
public static void writeToFile(String str1) {
System.out.print("Enter Text: ");
Scanner scan = new Scanner(System.in);
String text = scan.nextLine();
FileWriter fWriter = null;
BufferedWriter writer = null;
try {
fWriter = new FileWriter(str1);
writer = new BufferedWriter(fWriter);
writer.write(text);
writer.newLine();
writer.close();
System.err.println(
"Your input of " + text.length() + " characters was saved."
);
} catch (Exception e) {
System.out.println("Error!");
}
}
}
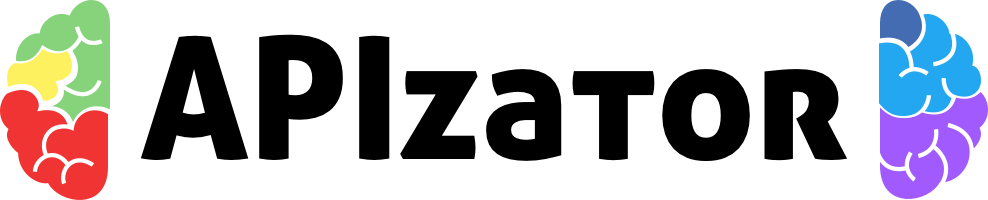
package com.stackoverflow.api;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.util.Scanner;
/**
* How to read from user's input in Java and write it to a file
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/18070669">https://stackoverflow.com/a/18070669</a>
*/
public class APIzator18070669 {
public static void read(String str1) {
System.out.print("Enter Text: ");
Scanner scan = new Scanner(System.in);
String text = scan.nextLine();
FileWriter fWriter = null;
BufferedWriter writer = null;
try {
fWriter = new FileWriter(str1);
writer = new BufferedWriter(fWriter);
writer.write(text);
writer.newLine();
writer.close();
System.err.println(
"Your input of " + text.length() + " characters was saved."
);
} catch (Exception e) {
System.out.println("Error!");
}
}
}