[Q#18119211][A#18119273] How to check if user input is not an int value
https://stackoverflow.com/q/18119211
I need to check if a user input value is not an int value. I've tried different combinations of what I know but I either get nothing or random errors For example: If the user inputs "adfadf 1324" it'll raise a warning message. What I have:
Answer
https://stackoverflow.com/a/18119273
Simply throw Exception if input is invalid
APIzation

Scanner sc=new Scanner(System.in);
try
{
System.out.println("Please input an integer");
//nextInt will throw InputMismatchException
//if the next token does not match the Integer
//regular expression, or is out of range
int usrInput=sc.nextInt();
}
catch(InputMismatchException exception)
{
//Print "This is not an integer"
//when user put other than integer
System.out.println("This is not an integer");
}
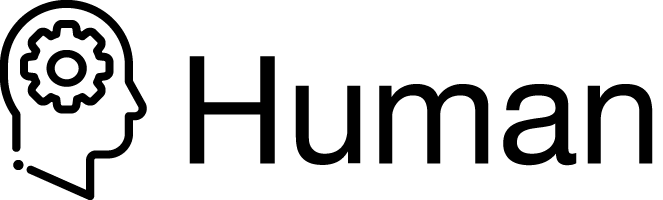
package com.stackoverflow.api;
import java.util.InputMismatchException;
import java.util.Scanner;
public class Human18119273 {
public static boolean userInputNotInt() {
Scanner sc = new Scanner(System.in);
try {
System.out.println("Please input an integer");
// nextInt will throw InputMismatchException
// if the next token does not match the Integer
// regular expression, or is out of range
sc.nextInt();
} catch (InputMismatchException exception) {
// Print "This is not an integer"
// when user put other than integer
System.out.println("This is not an integer");
sc.close();
return true;
}
sc.close();
return false;
}
}
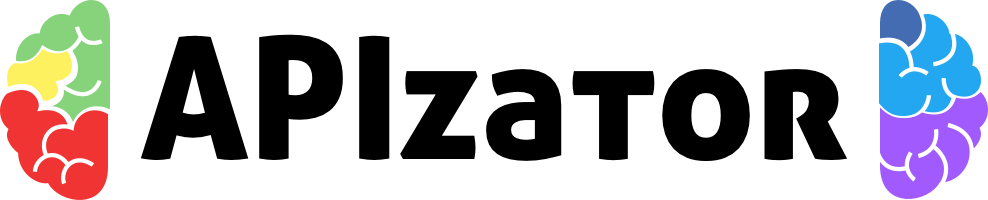
package com.stackoverflow.api;
import java.util.InputMismatchException;
import java.util.Scanner;
/**
* How to check if user input is not an int value
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/18119273">https://stackoverflow.com/a/18119273</a>
*/
public class APIzator18119273 {
public static void check() throws Exception {
Scanner sc = new Scanner(System.in);
try {
System.out.println("Please input an integer");
// nextInt will throw InputMismatchException
// if the next token does not match the Integer
// regular expression, or is out of range
int usrInput = sc.nextInt();
} catch (InputMismatchException exception) {
// Print "This is not an integer"
// when user put other than integer
System.out.println("This is not an integer");
}
}
}