[Q#18708087][A#18708275] How to execute bash command with sudo privileges in Java?
https://stackoverflow.com/q/18708087
I'm using ProcessBuilder to execute bash commands: But I want to make something like this: How to pass superuser password to bash? ("gksudo", "gedit") will not do the trick, because it was deleted since Ubuntu 13.04 and I need to do this with available by default commands. EDIT gksudo came back to Ubuntu 13.04 with the last update.
Answer
https://stackoverflow.com/a/18708275
I think you can use this, but I'm a bit hesitant to post it. So I'll just say: Use this at your own risk, not recommended, don't sue me, etc…
APIzation

public static void main(String[] args) throws IOException {
String[] cmd = {"/bin/bash","-c","echo password| sudo -S ls"};
Process pb = Runtime.getRuntime().exec(cmd);
String line;
BufferedReader input = new BufferedReader(new InputStreamReader(pb.getInputStream()));
while ((line = input.readLine()) != null) {
System.out.println(line);
}
input.close();
}
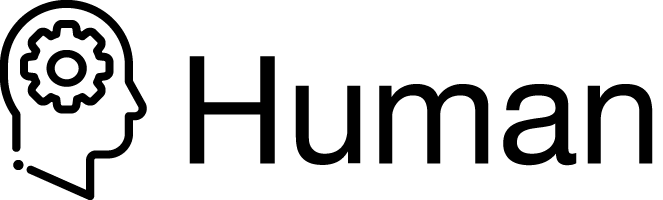
package com.stackoverflow.api;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Human18708275 {
public static void executeBashCommandWithSudoPrivileges() throws IOException {
String[] cmd = { "/bin/bash", "-c", "echo password| sudo -S ls" };
Process pb = Runtime.getRuntime().exec(cmd);
String line;
BufferedReader input = new BufferedReader(
new InputStreamReader(pb.getInputStream())
);
while ((line = input.readLine()) != null) {
System.out.println(line);
}
input.close();
}
}
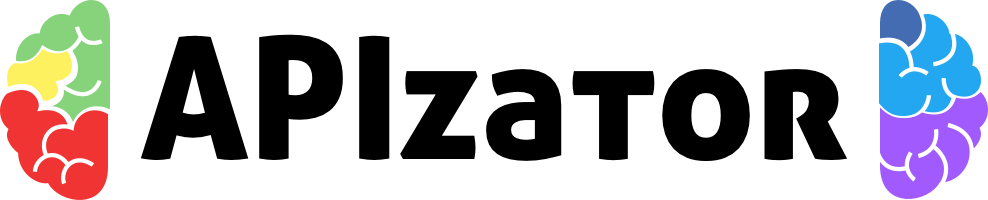
package com.stackoverflow.api;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
/**
* How to execute bash command with sudo privileges in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/18708275">https://stackoverflow.com/a/18708275</a>
*/
public class APIzator18708275 {
public static void executeCommand(String[] cmd) throws IOException {
Process pb = Runtime.getRuntime().exec(cmd);
String line;
BufferedReader input = new BufferedReader(
new InputStreamReader(pb.getInputStream())
);
while ((line = input.readLine()) != null) {
System.out.println(line);
}
input.close();
}
}