[Q#19066582][A#19066796] How to find vowels in a string in java program
https://stackoverflow.com/q/19066582
What is the java coding to find out vowels in a string? How to use OR operator in java? because the symbol " || " is not taken while i executing the java program.
Answer
https://stackoverflow.com/a/19066796
try the below code The program iterats over the given string and check if each character is an vowel
- The symbol '||' can be used as OR, below program is an example Note: it will match only lower case vowels
APIzation

public class Test {
public static void main(String[] args) {
String str ="This is a test";
for(int i=0;i <str.length();i++){
if((str.charAt(i) == 'a') ||
(str.charAt(i) == 'e') ||
(str.charAt(i) == 'i') ||
(str.charAt(i) == 'o') ||
(str.charAt(i) == 'u')) {
System.out.println(" The String contains " + str.charAt(i));
}
}
}
}
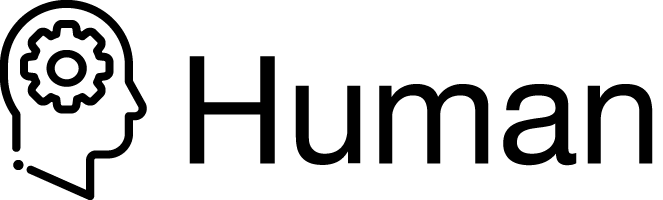
package com.stackoverflow.api;
public class Human19066796 {
/**
* Get lower case vowels from string
* @param str Input string
* @return Array of vowels
*/
public static char[] getVowelsFromString(String str) {
char[] vowels = new char[str.length()];
for (int i = 0; i < str.length(); i++) {
if (
(str.charAt(i) == 'a') ||
(str.charAt(i) == 'e') ||
(str.charAt(i) == 'i') ||
(str.charAt(i) == 'o') ||
(str.charAt(i) == 'u')
) {
vowels[vowels.length + 1] = str.charAt(i);
}
}
return vowels;
}
}
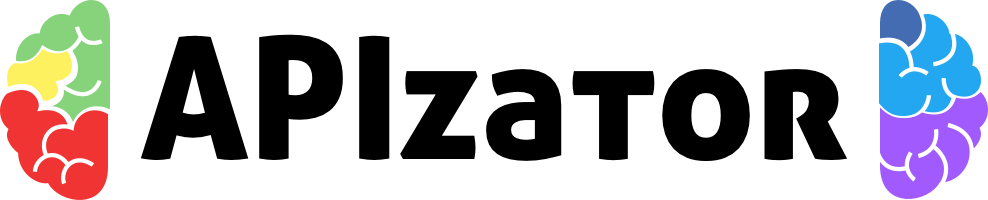
package com.stackoverflow.api;
/**
* How to find vowels in a string in java program
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/19066796">https://stackoverflow.com/a/19066796</a>
*/
public class APIzator19066796 {
public static void findVowel(String str) {
for (int i = 0; i < str.length(); i++) {
if (
(str.charAt(i) == 'a') ||
(str.charAt(i) == 'e') ||
(str.charAt(i) == 'i') ||
(str.charAt(i) == 'o') ||
(str.charAt(i) == 'u')
) {
System.out.println(" The String contains " + str.charAt(i));
}
}
}
}