[Q#19238065][A#19238077] How to convert char[] to string in java?
https://stackoverflow.com/q/19238065
char[] c = string.toCharArray(); but how to convert c back to String type? thank you!
Answer
https://stackoverflow.com/a/19238077
You can use String.valueOf(char[]): Under the hood, this calls the String(char[]) constructor. I always prefer factory-esque methods to constructors, but you could have used new String(c) just as easily, as several other answers have suggested.
APIzation

String.valueOf(c)
char[] c = {'x', 'y', 'z'};
String s = String.valueOf(c);
System.out.println(s);
xyz
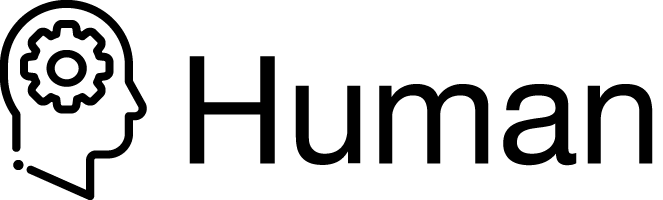
package com.stackoverflow.api;
public class Human19238077 {
public static String charArrayToString(char[] c) {
String s = String.valueOf(c);
return s;
}
}
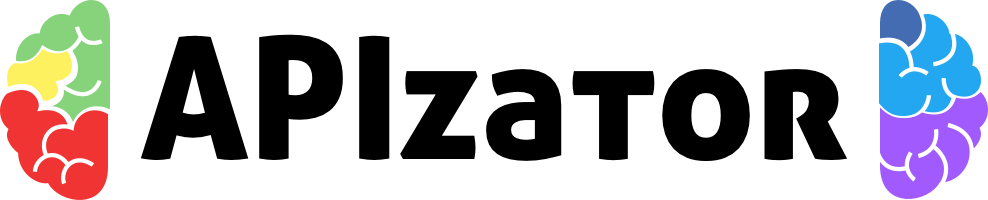
package com.stackoverflow.api;
/**
* How to convert char[] to string in java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/19238077">https://stackoverflow.com/a/19238077</a>
*/
public class APIzator19238077 {
public static String convertChar(char[] c) throws Exception {
String s = String.valueOf(c);
return s;
}
}