[Q#19452238][A#19452417] User will input some filter criteria -- how can I turn it into a regular expression for String.match
https://stackoverflow.com/q/19452238
I have a program where the user will enter a string such as PropertyA = "abc_*" and I need to have the asterisk match any character. In my code, I'm grabbing the property value and replacing PropertyA with the actual value. For instance, it could be abc_123. I also pull out the equality symbol into a variable. It should be able to cover this type of criteria PropertyB = 'cba' PropertyC != '*-this' valueFromHeader is the lefthand side and value is the righthand side. EDIT: The existing code had this type of replacement for regular expressions It doesn't like the underscore here… It doesn't like the underscore…
Answer
https://stackoverflow.com/a/19452417
Replace * with .*.. This won't work if your value contain +,? since they all have special meaning in regex.Escape them if their are any..
APIzation

value=value.replace("*",".*");//replace * with .*
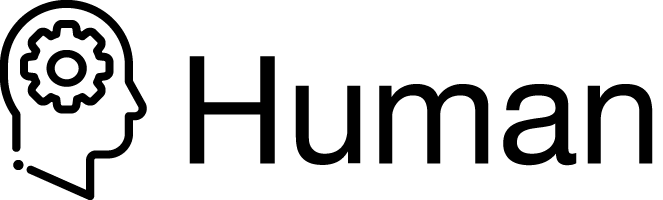
package com.stackoverflow.api;
public class Human19452417 {
public static String applyFilterCriteria(String value) {
final String ESC = "\\$1";
final String NON_ALPHA = "([^A-Za-z0-9@])";
final String WILD = "*";
final String WILD_RE_TEMP = "@";
final String WILD_RE = ".*";
value = value.replace(WILD, WILD_RE_TEMP);
value = value.replaceAll(NON_ALPHA, ESC);
value = value.replace(WILD_RE_TEMP, WILD_RE);
value = value.replace("*", ".*"); // replace * with .*
return value;
}
}
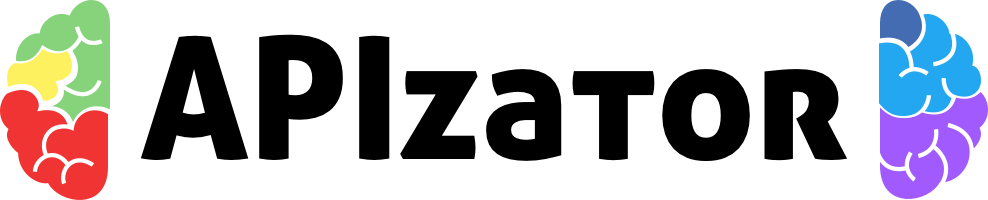
package com.stackoverflow.api;
/**
* User will input some filter criteria -- how can I turn it into a regular expression for String.match
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/19452417">https://stackoverflow.com/a/19452417</a>
*/
public class APIzator19452417 {
public static void inputCriterion(String value) throws Exception {
// replace * with .*
value = value.replace("*", ".*");
}
}