[Q#19751892][A#19751994] How to extract text between square brackets with String.split
https://stackoverflow.com/q/19751892
I have text which contains tags expressed in this format: [text other text]. I'd like to split the string using square brackets as separators, but this: This doesn't give expected results. How can I do this?
Answer
https://stackoverflow.com/a/19751994
I'm not sure if one can do this with split(). With pattern finding and [^\]]+ ("all symbols until the closing bracket") in your pattern this is quite straight-forward:
APIzation

public static void main(String[] args) {
String line = "xx [text other text], [jili u babusi dva veselikh gusya], " +
"[a granny there was having two gay gooses] zz";
Matcher matcher = Pattern.compile("\\[([^\\]]+)").matcher(line);
List<String> tags = new ArrayList<>();
int pos = -1;
while (matcher.find(pos+1)){
pos = matcher.start();
tags.add(matcher.group(1));
}
System.out.println(tags);
}
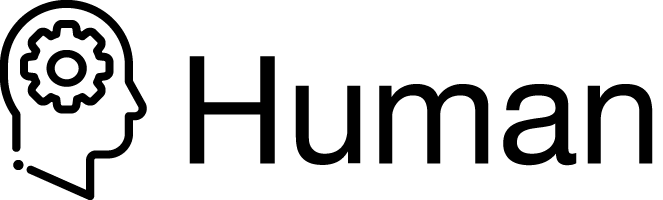
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human19751994 {
public static List<String> getGroupsFromString(String regex, String line) {
Matcher matcher = Pattern.compile(regex).matcher(line);
List<String> tags = new ArrayList<>();
int pos = -1;
while (matcher.find(pos + 1)) {
pos = matcher.start();
tags.add(matcher.group(1));
}
return tags;
}
}
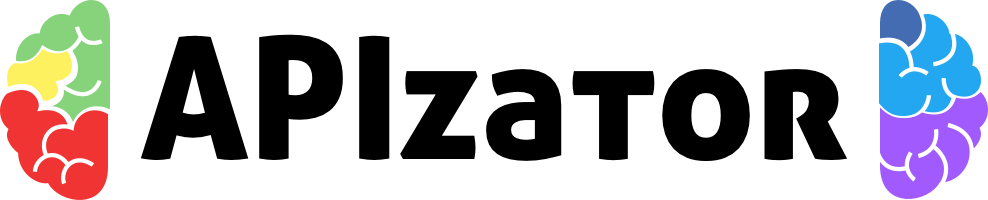
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How to extract text between square brackets with String.split
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/19751994">https://stackoverflow.com/a/19751994</a>
*/
public class APIzator19751994 {
public static List<String> extractText(String line) {
Matcher matcher = Pattern.compile("\\[([^\\]]+)").matcher(line);
List<String> tags = new ArrayList<>();
int pos = -1;
while (matcher.find(pos + 1)) {
pos = matcher.start();
tags.add(matcher.group(1));
}
return tags;
}
}