[Q#2015463][A#2015524] How to view the current heap size that an application is using?
https://stackoverflow.com/q/2015463
I think I increased my heap size to 1 GB in NetBeans since I changed the config to look like this: After I restarted NetBeans, can I be sure that my app is given 1 GB now? Is there a way to verify this?
Answer
https://stackoverflow.com/a/2015524
Use this code: It was useful to me to know it.
APIzation

// Get current size of heap in bytes
long heapSize = Runtime.getRuntime().totalMemory();
// Get maximum size of heap in bytes. The heap cannot grow beyond this size.// Any attempt will result in an OutOfMemoryException.
long heapMaxSize = Runtime.getRuntime().maxMemory();
// Get amount of free memory within the heap in bytes. This size will increase // after garbage collection and decrease as new objects are created.
long heapFreeSize = Runtime.getRuntime().freeMemory();
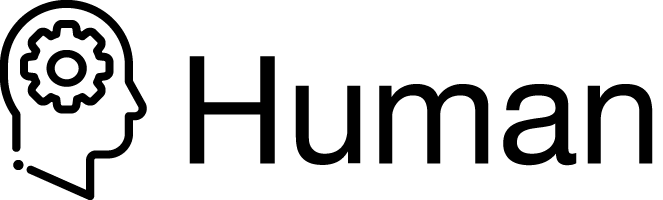
package com.stackoverflow.api;
public class Human2015524 {
public static MemoryInfo getMemoryInfo() {
// Get current size of heap in bytes
long heapSize = Runtime.getRuntime().totalMemory();
// Get maximum size of heap in bytes. The heap cannot grow beyond this size.// Any attempt will result in an OutOfMemoryException.
long heapMaxSize = Runtime.getRuntime().maxMemory();
// Get amount of free memory within the heap in bytes. This size will increase // after garbage collection and decrease as new objects are created.
long heapFreeSize = Runtime.getRuntime().freeMemory();
return new MemoryInfo(heapSize, heapMaxSize, heapFreeSize);
}
}
class MemoryInfo {
private long heapSize;
private long heapMaxSize;
private long heapFreeSize;
public MemoryInfo(long heapSize, long heapMaxSize, long heapFreeSize) {
this.heapSize = heapSize;
this.heapMaxSize = heapMaxSize;
this.heapFreeSize = heapFreeSize;
}
public long getHeapSize() {
return heapSize;
}
public long getHeapMaxSize() {
return heapMaxSize;
}
public long getHeapFreeSize() {
return heapFreeSize;
}
}
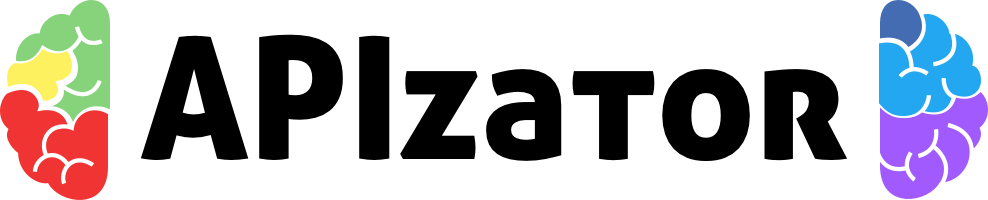
package com.stackoverflow.api;
/**
* How to view the current heap size that an application is using?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/2015524">https://stackoverflow.com/a/2015524</a>
*/
public class APIzator2015524 {
public static long viewSize() throws Exception {
// Get current size of heap in bytes
long heapSize = Runtime.getRuntime().totalMemory();
// Get maximum size of heap in bytes. The heap cannot grow beyond this size.// Any attempt will result in an OutOfMemoryException.
long heapMaxSize = Runtime.getRuntime().maxMemory();
return Runtime.getRuntime().freeMemory();
}
}