[Q#21438078][A#21438476] How to read char from standard input in Java
https://stackoverflow.com/q/21438078
I have to read char(one at a time) from the standard input in Java.The input will consist of numerous lines (each of about 10000 chars). I do not need to store the chars , they are processed when read.Also I need to skip the newline char .Can someone suggest me an efficient way to do this ?
Answer
https://stackoverflow.com/a/21438476
Perhaps something like this will work:
APIzation

import java.io.IOException;
public class Tester
{
public static void main(String args[])
throws IOException
{
int ch;
while ((ch = System.in.read()) != -1)
{
if (ch != '\n' && ch != '\r')
{
processChar((char)ch);
}
}
}
private static void processChar(char c)
{
// do stuff
System.out.println("Processing: '" + c + "'");
}
}
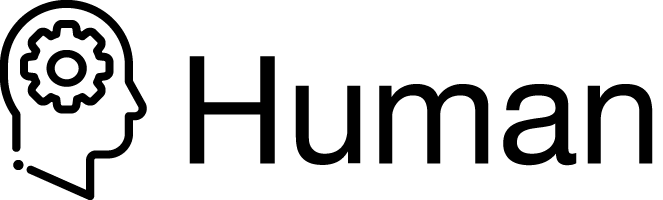
package com.stackoverflow.api;
import java.io.IOException;
public class Human21438476 {
public static void processStandardInput() throws IOException {
int ch;
while ((ch = System.in.read()) != -1) {
if (ch != '\n' && ch != '\r') {
processChar((char) ch);
}
}
}
private static void processChar(char c) {
// do stuff
System.out.println("Processing: '" + c + "'");
}
}
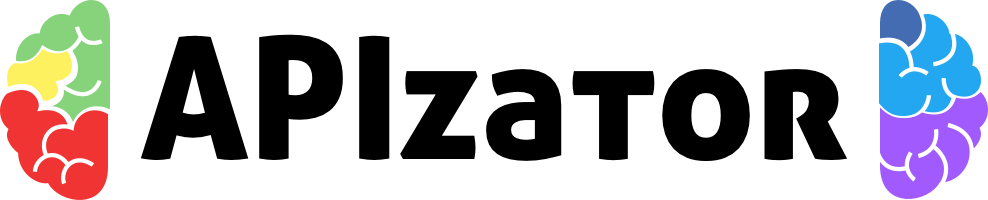
package com.stackoverflow.api;
import java.io.IOException;
/**
* How to read char from standard input in Java
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/21438476">https://stackoverflow.com/a/21438476</a>
*/
public class APIzator21438476 {
public static void readChar() throws IOException {
int ch;
while ((ch = System.in.read()) != -1) {
if (ch != '\n' && ch != '\r') {
processChar((char) ch);
}
}
}
private static void processChar(char c) {
// do stuff
System.out.println("Processing: '" + c + "'");
}
}