[Q#21625772][A#21625811] how to remove duplicate value from hash map
https://stackoverflow.com/q/21625772
This my code i want to remove all duplicate value from hash-map and want print on console please tell me how i will do this .
Answer
https://stackoverflow.com/a/21625811
Your HashMap is hm. Put the values of hm in another HashMap hm2 in which the values of hm are the keys of hm2, and the values of hm2 can be anything (e.g. the object Boolean.TRUE). Then loop through that second HashMap hm2 and print out its keys. Instead of HashMap you can also use HashSet for hm2 (this is even better as you would not need the Boolean.TRUE part).
APIzation

import java.util.HashMap;
import java.util.HashSet;
public class MyClass {
public static void main(String[] args) {
HashMap<Integer, String> hm = new HashMap<Integer, String>();
hm.put(1, "Anil");
hm.put(2, "Deven");
hm.put(3, "sanjay");
hm.put(4, "sanjay");
hm.put(5, "Raj");
hm.put(6, "sanjay");
HashSet<String> hm2 = new HashSet<String>();
hm2.addAll(hm.values());
for (String str : hm2){
System.out.println(str);
}
}
}
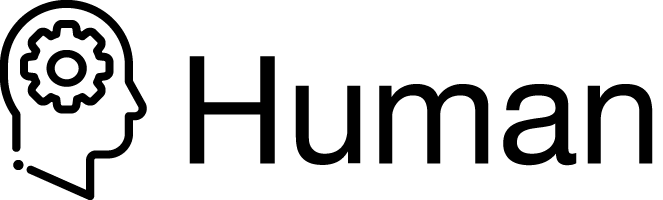
package com.stackoverflow.api;
import java.util.HashMap;
import java.util.HashSet;
public class Human21625811 {
public static HashSet<String> removeDuplicateValues(
HashMap<Integer, String> hm
) {
HashSet<String> hm2 = new HashSet<String>();
hm2.addAll(hm.values());
return hm2;
}
}
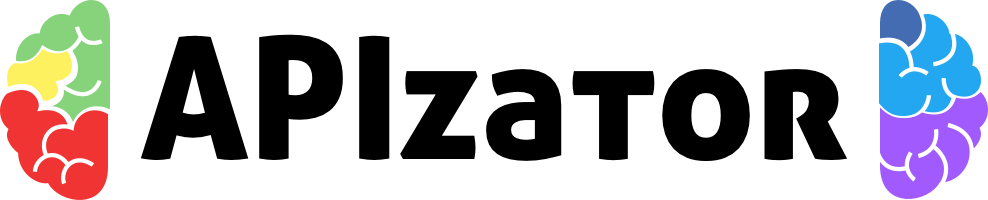
package com.stackoverflow.api;
import java.util.HashMap;
import java.util.HashSet;
/**
* how to remove duplicate value from hash map
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/21625811">https://stackoverflow.com/a/21625811</a>
*/
public class APIzator21625811 {
public static void removeValue(HashMap<Integer, String> hm) {
HashSet<String> hm2 = new HashSet<String>();
hm2.addAll(hm.values());
for (String str : hm2) {
System.out.println(str);
}
}
}