[Q#2318220][A#2318759] How to detect via Java whether a particular process is running under Windows?
https://stackoverflow.com/q/2318220
Well the title pretty much sums the question. The only thing I found is this but I'm not sure if thats the way to go.
Answer
https://stackoverflow.com/a/2318759
You can use the wmic utility to check the list of running processes. Suppose you want to check if the windows' explorer.exe process is running : See http://ss64.com/nt/wmic.html or http://support.microsoft.com/servicedesks/webcasts/wc072402/listofsampleusage.asp for some example of what you can get from wmic…
APIzation

String line;
try {
Process proc = Runtime.getRuntime().exec("wmic.exe");
BufferedReader input = new BufferedReader(new InputStreamReader(proc.getInputStream()));
OutputStreamWriter oStream = new OutputStreamWriter(proc.getOutputStream());
oStream .write("process where name='explorer.exe'");
oStream .flush();
oStream .close();
while ((line = input.readLine()) != null) {
System.out.println(line);
}
input.close();
} catch (IOException ioe) {
ioe.printStackTrace();
}
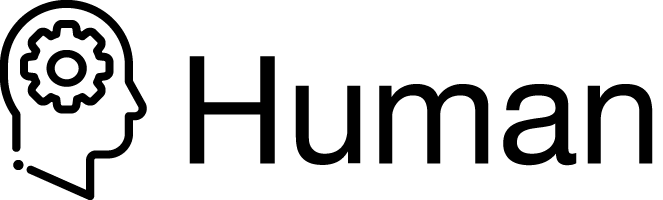
package com.stackoverflow.api;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
public class Human2318759 {
public static boolean checkProcess(String process) {
String line;
try {
Process proc = Runtime.getRuntime().exec("wmic.exe");
BufferedReader input = new BufferedReader(
new InputStreamReader(proc.getInputStream())
);
OutputStreamWriter oStream = new OutputStreamWriter(
proc.getOutputStream()
);
oStream.write("process where name='" + process + "'");
oStream.flush();
oStream.close();
while ((line = input.readLine()) != null) {
if (line.contains(process)) return true;
}
input.close();
} catch (IOException ioe) {
ioe.printStackTrace();
}
return false;
}
}
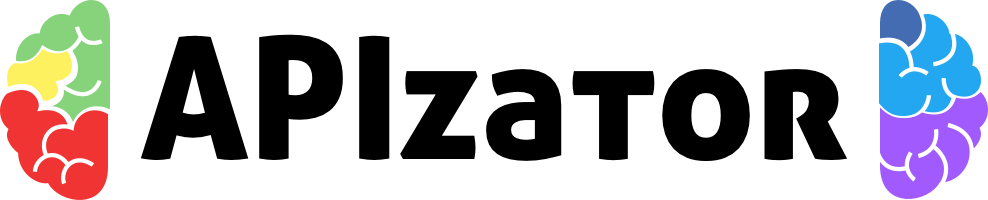
package com.stackoverflow.api;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
/**
* How to detect via Java whether a particular process is running under Windows?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/2318759">https://stackoverflow.com/a/2318759</a>
*/
public class APIzator2318759 {
public static void detect() throws Exception {
String line;
try {
Process proc = Runtime.getRuntime().exec("wmic.exe");
BufferedReader input = new BufferedReader(
new InputStreamReader(proc.getInputStream())
);
OutputStreamWriter oStream = new OutputStreamWriter(
proc.getOutputStream()
);
oStream.write("process where name='explorer.exe'");
oStream.flush();
oStream.close();
while ((line = input.readLine()) != null) {
System.out.println(line);
}
input.close();
} catch (IOException ioe) {
ioe.printStackTrace();
}
}
}