[Q#2367381][A#2367418] How to extract numbers from a string and get an array of ints?
https://stackoverflow.com/q/2367381
I have a String variable (basically an English sentence with an unspecified number of numbers) and I'd like to extract all the numbers into an array of integers. I was wondering whether there was a quick solution with regular expressions? I used Sean's solution and changed it slightly:
Answer
https://stackoverflow.com/a/2367418
… prints -2 and 12. -? matches a leading negative sign – optionally. \d matches a digit, and we need to write \ as \ in a Java String though. So, \d+ matches 1 or more digits.
APIzation

Pattern p = Pattern.compile("-?\\d+");
Matcher m = p.matcher("There are more than -2 and less than 12 numbers here");
while (m.find()) {
System.out.println(m.group());
}
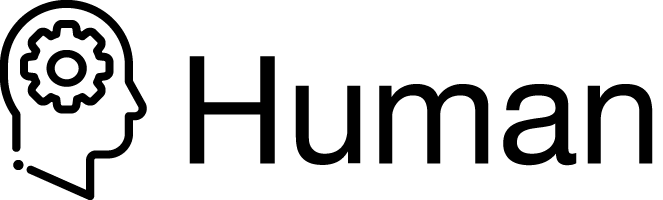
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human2367418 {
public static List<String> extractNumbersFromString(final String s) {
List<String> numbers = new ArrayList<>();
Pattern p = Pattern.compile("-?\\d+");
Matcher m = p.matcher(s);
while (m.find()) {
System.out.println(m.group());
numbers.add(m.group());
}
return numbers;
}
}
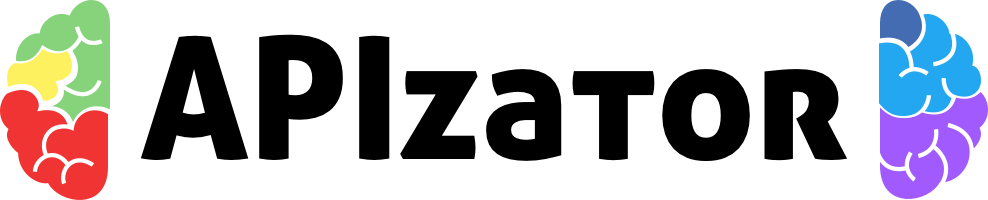
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How to extract numbers from a string and get an array of ints?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/2367418">https://stackoverflow.com/a/2367418</a>
*/
public class APIzator2367418 {
public static void extractNumber() throws Exception {
Pattern p = Pattern.compile("-?\\d+");
Matcher m = p.matcher(
"There are more than -2 and less than 12 numbers here"
);
while (m.find()) {
System.out.println(m.group());
}
}
}