[Q#2707357][A#2707431] how to create dynamic two dimensional array in java?
https://stackoverflow.com/q/2707357
I want to create a two dimensional array dynamically. I know the number of columns. But the number of rows are being changed dynamically. I tried the array list, but it stores the value in single dimension only. What can I do?
Answer
https://stackoverflow.com/a/2707431
Since the number of columns is a constant, you can just have an List of int[]. Since it's backed by a List, the number of rows can grow and shrink dynamically. Each row is backed by an int[], which is static, but you said that the number of columns is fixed, so this is not a problem.
APIzation

import java.util.*;
//...
List<int[]> rowList = new ArrayList<int[]>();
rowList.add(new int[] { 1, 2, 3 });
rowList.add(new int[] { 4, 5, 6 });
rowList.add(new int[] { 7, 8 });
for (int[] row : rowList) {
System.out.println("Row = " + Arrays.toString(row));
} // prints:
// Row = [1, 2, 3]
// Row = [4, 5, 6]
// Row = [7, 8]
System.out.println(rowList.get(1)[1]); // prints "5"
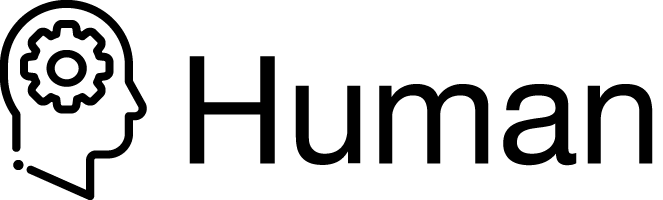
package com.stackoverflow.api;
import java.util.ArrayList;
import java.util.List;
public class Human2707431 {
public static List<int[]> create2DArray(
int[] array,
int[] array1,
int[] array2
) {
List<int[]> rowList = new ArrayList<>();
rowList.add(array);
rowList.add(array1);
rowList.add(array2);
return rowList;
}
}
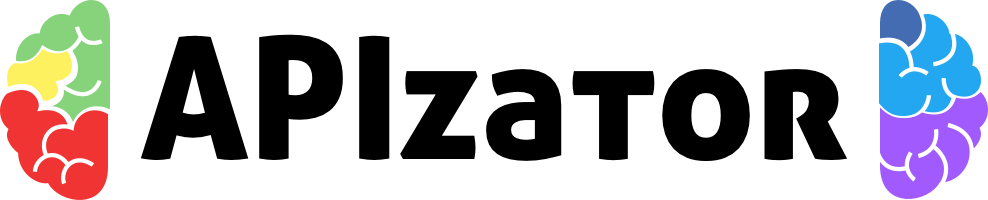
package com.stackoverflow.api;
import java.util.*;
/**
* how to create dynamic two dimensional array in java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/2707431">https://stackoverflow.com/a/2707431</a>
*/
public class APIzator2707431 {
public static void createArray(List<int[]> rowList) throws Exception {
// ...
for (int[] row : rowList) {
System.out.println("Row = " + Arrays.toString(row));
}
// prints:
// Row = [1, 2, 3]
// Row = [4, 5, 6]
// Row = [7, 8]
// prints "5"
System.out.println(rowList.get(1)[1]);
}
}