[Q#27096670][A#27096697] How to sum digits of an integer in java?
https://stackoverflow.com/q/27096670
I am having a hard time figuring out the solution to this problem. I am trying to develop a program in Java that takes a number, such as 321, and finds the sum of digits, in this case 3 + 2 + 1 = 6. I need all the digits of any three digit number to add them together, and store that value using the % remainder symbol. This has been confusing me and I would appreciate anyones ideas.
Answer
https://stackoverflow.com/a/27096697
Output
APIzation

public static void main(String[] args) {
int num = 321;
int sum = 0;
while (num > 0) {
sum = sum + num % 10;
num = num / 10;
}
System.out.println(sum);
}
6
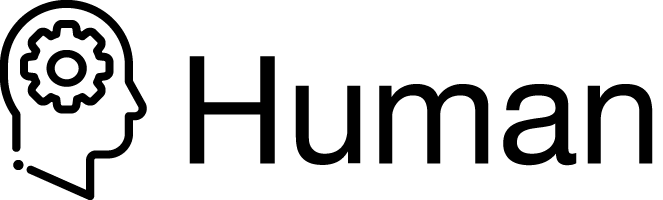
package com.stackoverflow.api;
public class Human27096697 {
public static int sumDigits(int num) {
int sum = 0;
while (num > 0) {
sum = sum + num % 10;
num = num / 10;
}
return sum;
}
}
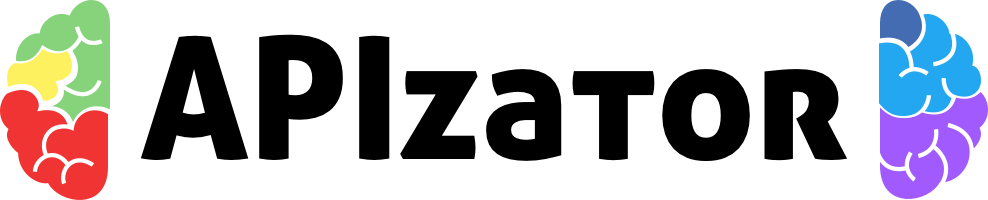
package com.stackoverflow.api;
/**
* How to sum digits of an integer in java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/27096697">https://stackoverflow.com/a/27096697</a>
*/
public class APIzator27096697 {
public static int sumDigit() {
int num = 321;
int sum = 0;
while (num > 0) {
sum = sum + num % 10;
num = num / 10;
}
return sum;
}
}