[Q#28728147][A#28728429] How can I split a string based on chaging characters rather than one single separator?
https://stackoverflow.com/q/28728147
I'm looking for a solution in java that will allow me to split a String like this one "MM/dd/yyyy", not based on the "/" slash but based on the change from "M" to "d" and from "d" to "y"? The reason is that I may also need to split a string using this logic that has no separator at all, for example "yyMMdd". Note: I'm looking for splitting the pattern string itself, and not some actual date string which follows those patterns. Any ideas?
Answer
https://stackoverflow.com/a/28728429
Given that you just want to split pattern - yy/MM/dd or any other, and not an actual date formatted to those patterns, I would rather use Matcher here, instead of split(). This solution rather than splitting on some unknown delimiter, just finds a sequence of y or M or d (As in any pattern, those will be coming in sequence only).
APIzation

String patternString = "yy/MM/dd";
Pattern pattern = Pattern.compile("y+|M+|d+");
Matcher matcher = pattern.matcher(patternString);
while (matcher.find()) {
System.out.println(matcher.group());
}
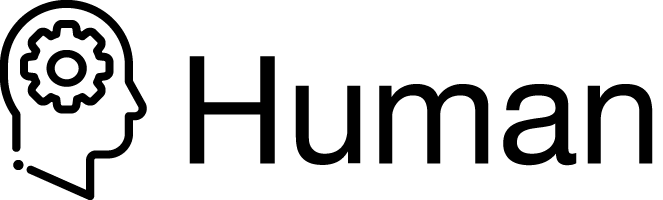
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human28728429 {
public static void splitPatterns(String p, String patternString) {
Pattern pattern = Pattern.compile(p);
Matcher matcher = pattern.matcher(patternString);
while (matcher.find()) {
System.out.println(matcher.group());
}
}
}
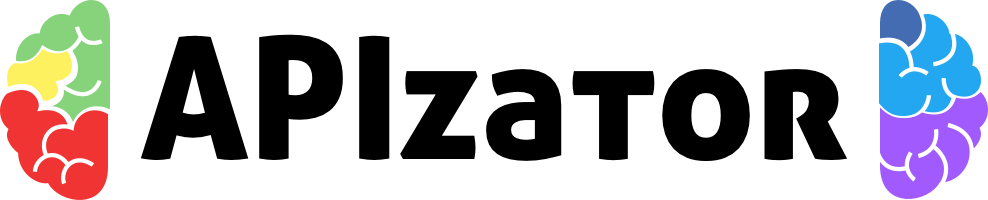
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How can I split a string based on chaging characters rather than one single separator?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/28728429">https://stackoverflow.com/a/28728429</a>
*/
public class APIzator28728429 {
public static void splitString(String patternString) throws Exception {
Pattern pattern = Pattern.compile("y+|M+|d+");
Matcher matcher = pattern.matcher(patternString);
while (matcher.find()) {
System.out.println(matcher.group());
}
}
}