[Q#3035880][A#3035893] How can I create a bar in the bottom of a Java app, like a status bar?
https://stackoverflow.com/q/3035880
I am in the process of creating a Java app and would like to have a bar on the bottom of the app, in which I display a text bar and a status (progress) bar. Only I can't seem to find the control in NetBeans neither do I know the code to create in manually.
Answer
https://stackoverflow.com/a/3035893
Create a JFrame or JPanel with a BorderLayout, give it something like a BevelBorder or line border so it is seperated off from the rest of the content and then add the status panel at BorderLayout.SOUTH. Here is the result of the above status bar code on my machine:
APIzation

JFrame frame = new JFrame();
frame.setLayout(new BorderLayout());
frame.setSize(200, 200);
// create the status bar panel and shove it down the bottom of the frame
JPanel statusPanel = new JPanel();
statusPanel.setBorder(new BevelBorder(BevelBorder.LOWERED));
frame.add(statusPanel, BorderLayout.SOUTH);
statusPanel.setPreferredSize(new Dimension(frame.getWidth(), 16));
statusPanel.setLayout(new BoxLayout(statusPanel, BoxLayout.X_AXIS));
JLabel statusLabel = new JLabel("status");
statusLabel.setHorizontalAlignment(SwingConstants.LEFT);
statusPanel.add(statusLabel);
frame.setVisible(true);
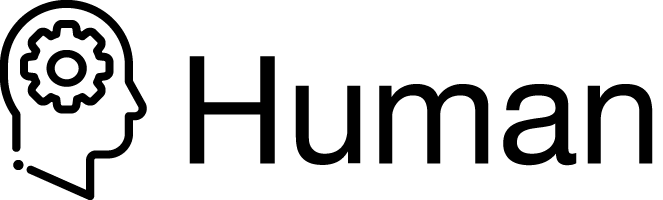
package com.stackoverflow.api;
import java.awt.*;
import javax.swing.*;
import javax.swing.border.BevelBorder;
public class Human3035893 {
public static JFrame createBar(String text) {
JFrame frame = new JFrame();
frame.setLayout(new BorderLayout());
frame.setSize(200, 200);
// create the status bar panel and shove it down the bottom of the frame
JPanel statusPanel = new JPanel();
statusPanel.setBorder(new BevelBorder(BevelBorder.LOWERED));
frame.add(statusPanel, BorderLayout.SOUTH);
statusPanel.setPreferredSize(new Dimension(frame.getWidth(), 16));
statusPanel.setLayout(new BoxLayout(statusPanel, BoxLayout.X_AXIS));
JLabel statusLabel = new JLabel(text);
statusLabel.setHorizontalAlignment(SwingConstants.LEFT);
statusPanel.add(statusLabel);
frame.setVisible(true);
return frame;
}
}
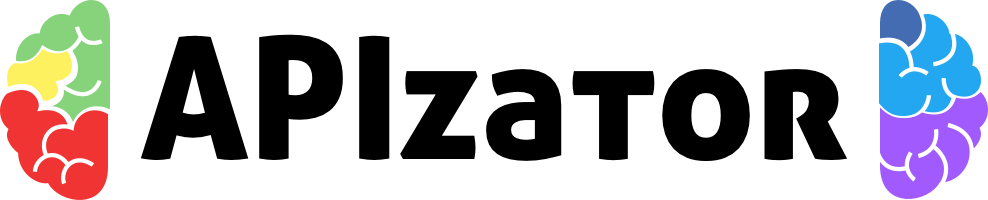
package com.stackoverflow.api;
import java.awt.BorderLayout;
import java.awt.Dimension;
import javax.swing.BoxLayout;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.border.BevelBorder;
/**
* How can I create a bar in the bottom of a Java app, like a status bar?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/3035893">https://stackoverflow.com/a/3035893</a>
*/
public class APIzator3035893 {
public static void createBar() throws Exception {
JFrame frame = new JFrame();
frame.setLayout(new BorderLayout());
frame.setSize(200, 200);
// create the status bar panel and shove it down the bottom of the frame
JPanel statusPanel = new JPanel();
statusPanel.setBorder(new BevelBorder(BevelBorder.LOWERED));
frame.add(statusPanel, BorderLayout.SOUTH);
statusPanel.setPreferredSize(new Dimension(frame.getWidth(), 16));
statusPanel.setLayout(new BoxLayout(statusPanel, BoxLayout.X_AXIS));
JLabel statusLabel = new JLabel("status");
statusLabel.setHorizontalAlignment(SwingConstants.LEFT);
statusPanel.add(statusLabel);
frame.setVisible(true);
}
}