[Q#31806686][A#31807319] How to execute Symlink command in java
https://stackoverflow.com/q/31806686
I am finding trouble in executing symlink command in java. My source filename and destination filename has space in it. How to execute this command? Eg. ln -sf /home/Desktop/image1 .jpg /home/Desktop/Folder/image 2.jpg I am trying this code
Answer
https://stackoverflow.com/a/31807319
You can do it easy with NIO.2
APIzation

Path directoryTarget = Paths.get("c:/temp");
Path directoryLink = Paths.get("c:/links/linkTemp");
Files.exists(directoryTarget);
try {
Files.createSymbolicLink(directoryLink, directoryTarget);
} catch (IOException e) {
e.printStackTrace();
}
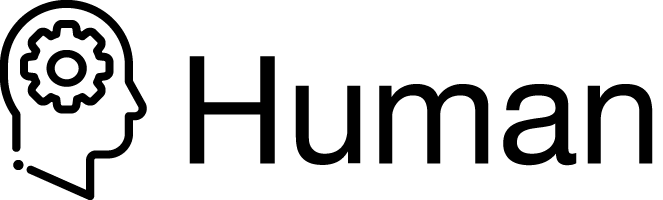
package com.stackoverflow.api;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
public class Human31807319 {
public static void createSymlink(Path directoryLink, Path directoryTarget) {
Files.exists(directoryTarget);
try {
Files.createSymbolicLink(directoryLink, directoryTarget);
} catch (IOException e) {
e.printStackTrace();
}
}
}
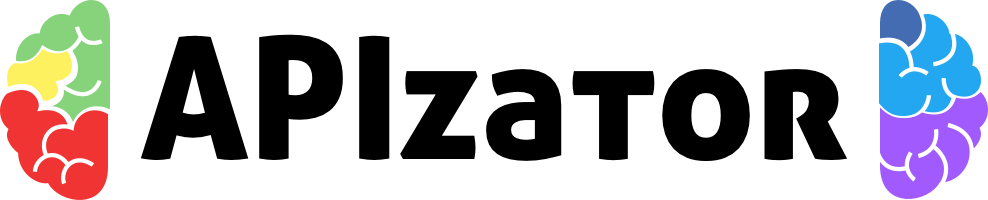
package com.stackoverflow.api;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
/**
* How to execute Symlink command in java
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/31807319">https://stackoverflow.com/a/31807319</a>
*/
public class APIzator31807319 {
public static void executeCommand() throws Exception {
Path directoryTarget = Paths.get("c:/temp");
Path directoryLink = Paths.get("c:/links/linkTemp");
Files.exists(directoryTarget);
try {
Files.createSymbolicLink(directoryLink, directoryTarget);
} catch (IOException e) {
e.printStackTrace();
}
}
}