[Q#32361477][A#32362085] How do I check if there's a time inside a string?
https://stackoverflow.com/q/32361477
I'm trying to check if a String contains a time, 12 or 24 hour format, using this regex: .([01]?[0-9]|2[0-3]):[0-5][0-9]. and used String.matches(), but it didn't seem to work. Am I doing something wrong? Also, I know String.matches() doesn't work the same way String.contains() but I read that adding a .* at the beginning and end of the regex makes it act that way from here. I would really appreciate it if someone could help me with this! EDIT: An example in which I'm trying to check if a time exists in a String would be like, "The current time in London is 6:00 PM, what is the time in Toronto?", and it would return true because 6:00 PM is inside the String.
Answer
https://stackoverflow.com/a/32362085
I used Pattern and Matcher for this answer…. Sample outputs…
APIzation

import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class IsTimeInString {
public static void main(String args[]){
String string = args[0];
System.out.println(String.format("Is there a time in here:(%1$s)", string));
Pattern p = Pattern.compile(".*([01]?[0-9]|2[0-3]):[0-5][0-9].*");
Matcher m = p.matcher(string);
if(m.matches()){
System.out.println("Yes");
}else{
System.out.println("No.");
}
}
}
$ java IsTimeInString "hi there"
Is there a time in here:(hi there)
No.
$ java IsTimeInString "hi there 2:15"
Is there a time in here:(hi there 2:15)
Yes
$ java IsTimeInString "hi there 14:15"
Is there a time in here:(hi there 14:15)
Yes
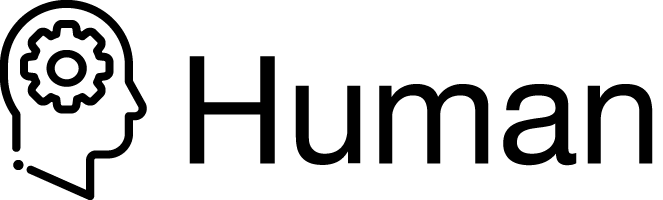
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human32362085 {
public static boolean timeInString(String str1) {
String string = str1;
System.out.println(String.format("Is there a time in here:(%1$s)", string));
Pattern p = Pattern.compile(".*([01]?[0-9]|2[0-3]):[0-5][0-9].*");
Matcher m = p.matcher(string);
if (m.matches()) {
return true;
} else {
return false;
}
}
}
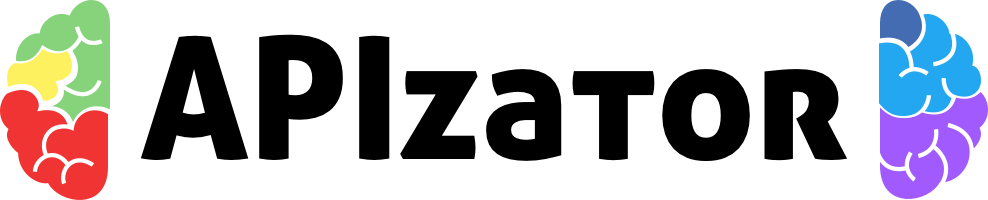
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How do I check if there's a time inside a string?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/32362085">https://stackoverflow.com/a/32362085</a>
*/
public class APIzator32362085 {
public static void check(String str1) {
String string = str1;
System.out.println(String.format("Is there a time in here:(%1$s)", string));
Pattern p = Pattern.compile(".*([01]?[0-9]|2[0-3]):[0-5][0-9].*");
Matcher m = p.matcher(string);
if (m.matches()) {
System.out.println("Yes");
} else {
System.out.println("No.");
}
}
}