[Q#35499385][A#35499670] How can I compare two strings and return the lexicographical ordered result using the compareTo method?
https://stackoverflow.com/q/35499385
I am trying to use compareTo method to compare two different names. After running the first attempt the program terminates immediately without returning anything. How can I modify this compareTo method to compare the names (Name n and Name n2) and return the result (-1, 1 or 0)? And obviously a print statement can be added to display (equal, before , or after) for the comparison. Thanks for any assistance.
Answer
https://stackoverflow.com/a/35499670
Outoput: By the way, check this out because every programming language has its own set of rules and conventions and for variables in Java is like this: If the name you choose consists of only one word, spell that word in all lowercase letters. If it consists of more than one word, capitalize the first letter of each subsequent word.
APIzation

//First attempt
public class Name {
public static void main(String[] args) {
String n = new String("jennifer");
String n2 = new String("paul");
if (n.compareTo(n2) < 0) {
System.out.println(n +" is before than " +n2);
} else if (n.compareTo(n2) > 0) {
System.out.println(n +" is after than " +n2);
} else if (n.compareTo(n2) == 0) {
System.out.println(n +" is equals to " +n);
}
}
}
jennifer is before than paul
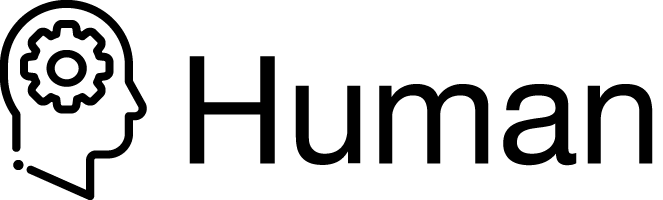
package com.stackoverflow.api;
public class Human35499670 {
public static void compareStrings(String first, String second) {
String n = first;
String n2 = second;
if (n.compareTo(n2) < 0) {
System.out.println(n + " is before than " + n2);
} else if (n.compareTo(n2) > 0) {
System.out.println(n + " is after than " + n2);
} else if (n.compareTo(n2) == 0) {
System.out.println(n + " is equals to " + n);
}
}
}
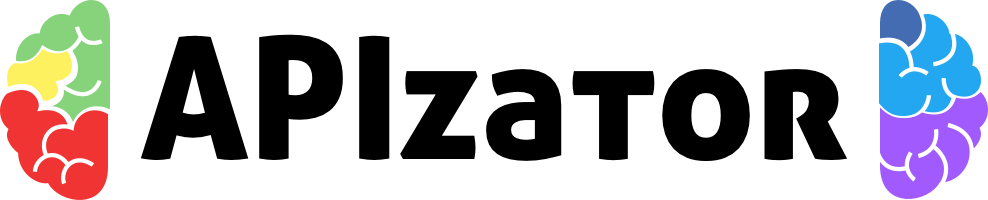
package com.stackoverflow.api;
/**
* How can I compare two strings and return the lexicographical ordered result using the compareTo method?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/35499670">https://stackoverflow.com/a/35499670</a>
*/
public class APIzator35499670 {
public static void compareString() {
String n = new String("jennifer");
String n2 = new String("paul");
if (n.compareTo(n2) < 0) {
System.out.println(n + " is before than " + n2);
} else if (n.compareTo(n2) > 0) {
System.out.println(n + " is after than " + n2);
} else if (n.compareTo(n2) == 0) {
System.out.println(n + " is equals to " + n);
}
}
}