[Q#36568590][A#36568643] How to create a sequence of numbers in java
https://stackoverflow.com/q/36568590
I want to create a sequence of numbers in java like this : I want to create 1000 numbers in the sequence .. How can I do it? I tried to do like this : it does not work , it prints out : 999 thanks
Answer
https://stackoverflow.com/a/36568643
As a general note, while str = str + someString; will work, inside a loop it can quickly get out of hand. Try it with 10000 iterations and you'll see (large amounts of RAM & CPU consumed). StringBuilder is better, if one really needs to build a string in this way, and it's always better performace-wise when one is appending to a character sequence more than a couple of times.
APIzation

StringBuilder sb = new StringBuilder();
for (int i=0; i<1000; i++) {
sb.append(i);
}
System.out.println(sb.toString());
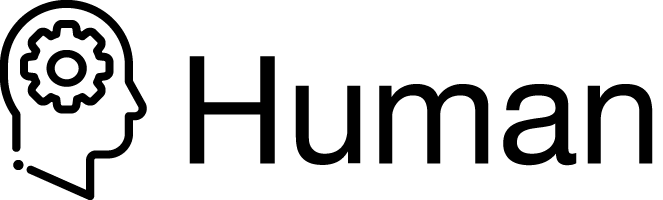
package com.stackoverflow.api;
public class Human36568643 {
public static String numberSequence() {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append(i);
}
return sb.toString();
}
}
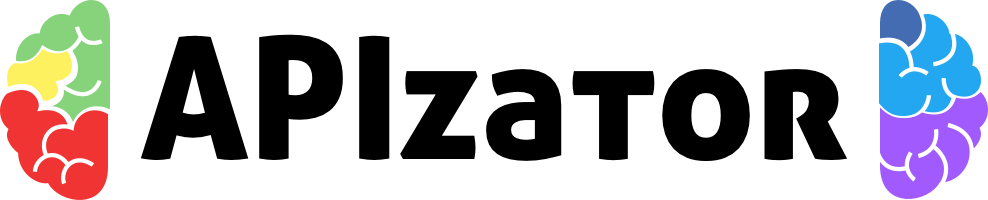
package com.stackoverflow.api;
/**
* How to create a sequence of numbers in java
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/36568643">https://stackoverflow.com/a/36568643</a>
*/
public class APIzator36568643 {
public static String createSequence() throws Exception {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append(i);
}
return sb.toString();
}
}