[Q#3870847][A#3871163] How to convert the DataInputStream to the String in Java?
https://stackoverflow.com/q/3870847
I want to ask a question about Java. I have use the URLConnection in Java to retrieve the DataInputStream. and I want to convert the DataInputStream into a String variable in Java. What should I do? Can anyone help me. thank you. The following is my code:
Answer
https://stackoverflow.com/a/3871163
This is what you want.
APIzation

import java.net.*;
import java.io.*;
class ConnectionTest {
public static void main(String[] args) {
try {
URL google = new URL("http://www.google.com/");
URLConnection googleConnection = google.openConnection();
DataInputStream dis = new DataInputStream(googleConnection.getInputStream());
StringBuffer inputLine = new StringBuffer();
String tmp;
while ((tmp = dis.readLine()) != null) {
inputLine.append(tmp);
System.out.println(tmp);
}
//use inputLine.toString(); here it would have whole source
dis.close();
} catch (MalformedURLException me) {
System.out.println("MalformedURLException: " + me);
} catch (IOException ioe) {
System.out.println("IOException: " + ioe);
}
}
}
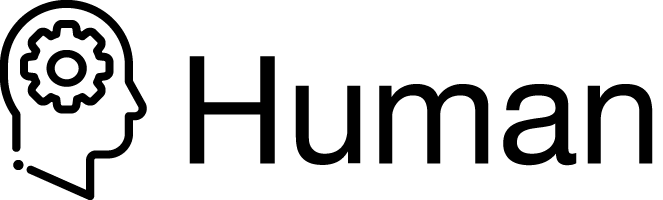
package com.stackoverflow.api;
import java.io.*;
import java.net.*;
public class Human3871163 {
public static String dataInputStream2String(DataInputStream dis) {
String tmp = null;
try {
StringBuffer inputLine = new StringBuffer();
while ((tmp = dis.readLine()) != null) {
inputLine.append(tmp);
}
//use inputLine.toString(); here it would have whole source
dis.close();
} catch (MalformedURLException me) {
System.out.println("MalformedURLException: " + me);
} catch (IOException ioe) {
System.out.println("IOException: " + ioe);
}
return tmp;
}
}
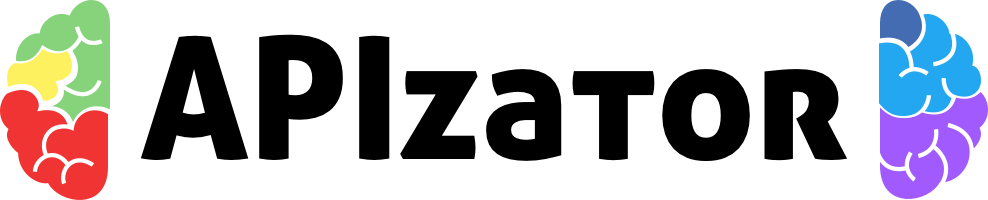
package com.stackoverflow.api;
import java.io.*;
import java.net.*;
/**
* How to convert the DataInputStream to the String in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/3871163">https://stackoverflow.com/a/3871163</a>
*/
public class APIzator3871163 {
public static void convertDatainputstream() {
try {
URL google = new URL("http://www.google.com/");
URLConnection googleConnection = google.openConnection();
DataInputStream dis = new DataInputStream(
googleConnection.getInputStream()
);
StringBuffer inputLine = new StringBuffer();
String tmp;
while ((tmp = dis.readLine()) != null) {
inputLine.append(tmp);
System.out.println(tmp);
}
// use inputLine.toString(); here it would have whole source
dis.close();
} catch (MalformedURLException me) {
System.out.println("MalformedURLException: " + me);
} catch (IOException ioe) {
System.out.println("IOException: " + ioe);
}
}
}