[Q#40910779][A#40910835] How to remove all characters before a specific character in Java?
https://stackoverflow.com/q/40910779
I have a string and I'm getting value through a html form so when I get the value it comes in a URL so I want to remove all the characters before the specific charater which is = and I also want to remove this character. I only want to save the value that comes after = because I need to fetch that value from the variable.. EDIT : I need to remove the = too since I'm trying to get the characters/value in string after it…
Answer
https://stackoverflow.com/a/40910835
You can use .substring(): then s1 contains everything after = in the original string. .trim() removes spaces before the first character (which isn't a whitespace, such as letters, numbers etc.) of a string (leading spaces) and also removes spaces after the last character (trailing spaces).
APIzation

String s = "the text=text";
String s1 = s.substring(s.indexOf("=")+1);
s1.trim();
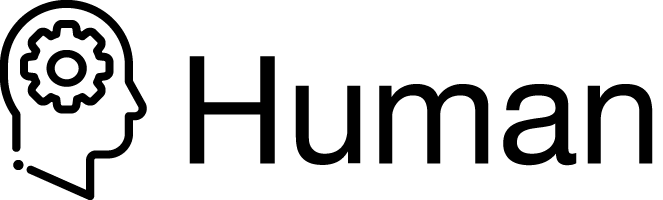
package com.stackoverflow.api;
public class Human40910835 {
public static String removeAllCharactersBefore(String s, char c) {
String s1 = s.substring(s.indexOf(c) + 1);
return s1.trim();
}
}
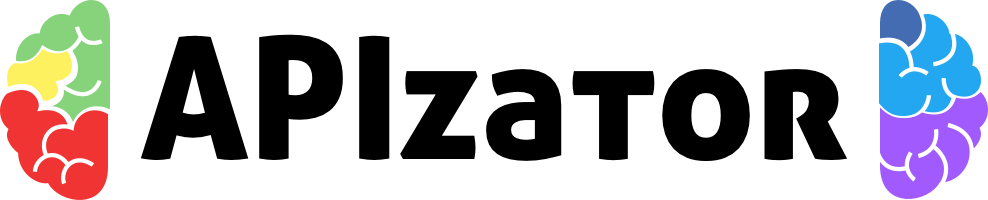
package com.stackoverflow.api;
/**
* How to remove all characters before a specific character in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/40910835">https://stackoverflow.com/a/40910835</a>
*/
public class APIzator40910835 {
public static void removeCharacter(String s) throws Exception {
String s1 = s.substring(s.indexOf("=") + 1);
s1.trim();
}
}