[Q#4385392][A#4385419] How to connect Java to Mysql?
https://stackoverflow.com/q/4385392
I got these errors for my Java program. I have already put the mysql-connector-java-5.1.14-bin.jar inside my classpath. How to solve this? The code:
Answer
https://stackoverflow.com/a/4385419
You need to download the mysql package from: here and place it inside the library, i'll edit the excact steps in a few min this is the correct syntax to connect to a database: Hope this helps
APIzation

try
{
// create a java mysql database connection
String myDriver = "org.gjt.mm.mysql.Driver";
String myUrl = "jdbc:mysql://localhost/test";
Class.forName(myDriver);
Connection conn = DriverManager.getConnection(myUrl, "root", "");
// your prepstatements goes here...
conn.close();
}
catch (Exception e)
{
System.err.println("Got an exception! ");
System.err.println(e.getMessage());
}
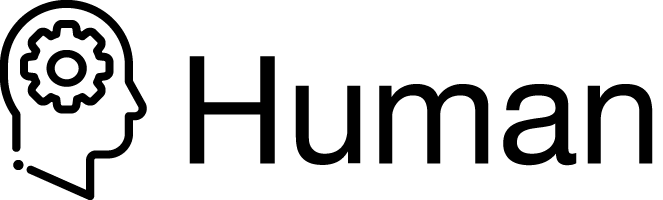
package com.stackoverflow.api;
import java.sql.Connection;
import java.sql.DriverManager;
public class Human4385419 {
public static Connection connectMySql(
String myUrl,
String user,
String password
) {
Connection conn = null;
try {
// create a java mysql database connection
String myDriver = "org.gjt.mm.mysql.Driver";
Class.forName(myDriver);
conn = DriverManager.getConnection(myUrl, user, password);
} catch (Exception e) {
System.err.println("Got an exception! ");
System.err.println(e.getMessage());
}
return conn;
}
}
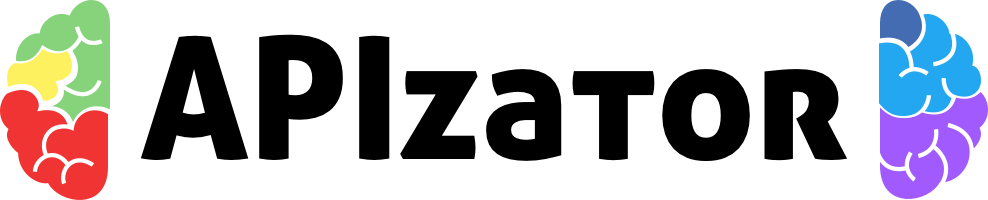
package com.stackoverflow.api;
import java.sql.Connection;
import java.sql.DriverManager;
/**
* How to connect Java to Mysql?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/4385419">https://stackoverflow.com/a/4385419</a>
*/
public class APIzator4385419 {
public static void connectJava(String myDriver, String myUrl)
throws Exception {
try {
Class.forName(myDriver);
Connection conn = DriverManager.getConnection(myUrl, "root", "");
// your prepstatements goes here...
conn.close();
} catch (Exception e) {
System.err.println("Got an exception! ");
System.err.println(e.getMessage());
}
}
}