[Q#4499562][A#4499595] How to save the data into File in java?
https://stackoverflow.com/q/4499562
I have one problem, that is I have one string of data and I want to save it into a separate file every time. Please give me a suggestion. Thanks, vara kumar.pjd
Answer
https://stackoverflow.com/a/4499595
Use a timestamp in de filename so you can be sure it is unique. This is something that is very easy to google.
APIzation

import java.io.*;
class FileWrite
{
public static void main(String args[])
{
try{
// Create file
FileWriter fstream = new FileWriter(System.currentTimeMillis() + "out.txt");
BufferedWriter out = new BufferedWriter(fstream);
out.write("Hello Java");
//Close the output stream
out.close();
}catch (Exception e){//Catch exception if any
System.err.println("Error: " + e.getMessage());
}
}
}
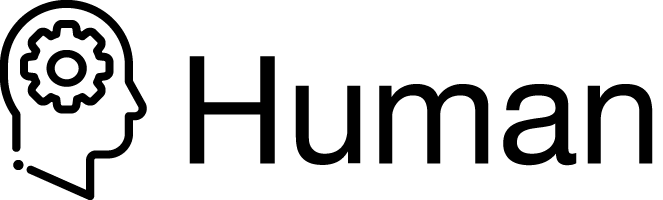
package com.stackoverflow.api;
import java.io.BufferedWriter;
import java.io.FileWriter;
public class Human4499595 {
public static void test(String fileName, String output) {
try {
FileWriter fstream = new FileWriter(fileName);
BufferedWriter out = new BufferedWriter(fstream);
out.write(output);
out.close();
} catch (Exception e) {
System.err.println("Error: " + e.getMessage());
}
}
}
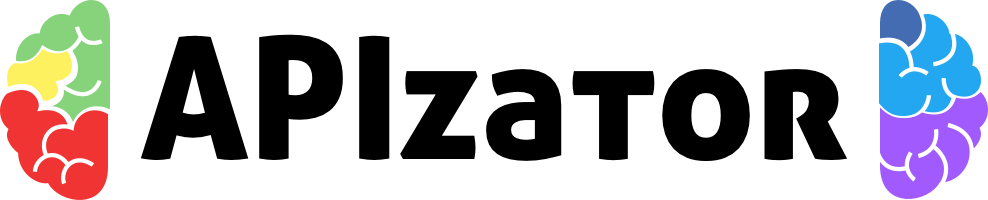
package com.stackoverflow.api;
import java.io.*;
/**
* How to save the data into File in java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/4499595">https://stackoverflow.com/a/4499595</a>
*/
public class APIzator4499595 {
public static void saveDatum() {
try {
// Create file
FileWriter fstream = new FileWriter(
System.currentTimeMillis() + "out.txt"
);
BufferedWriter out = new BufferedWriter(fstream);
out.write("Hello Java");
// Close the output stream
out.close();
} catch (Exception e) {
// Catch exception if any
System.err.println("Error: " + e.getMessage());
}
}
}