[Q#4662215][A#4662265] How to extract a substring using regex
https://stackoverflow.com/q/4662215
I have a string that has two single quotes in it, the ' character. In between the single quotes is the data I want. How can I write a regex to extract "the data i want" from the following text?
Answer
https://stackoverflow.com/a/4662265
Assuming you want the part between single quotes, use this regular expression with a Matcher: Example: Result:
APIzation

"'(.*?)'"
String mydata = "some string with 'the data i want' inside";
Pattern pattern = Pattern.compile("'(.*?)'");
Matcher matcher = pattern.matcher(mydata);
if (matcher.find())
{
System.out.println(matcher.group(1));
}
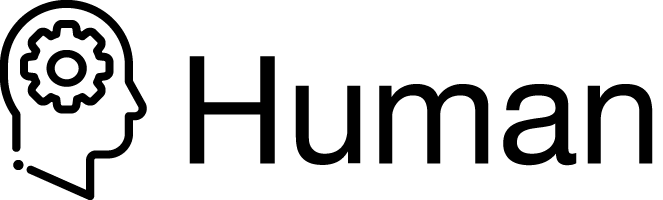
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human4662265 {
public static String extractFromSingleQuotes(String mydata) {
Pattern pattern = Pattern.compile("'(.*?)'");
Matcher matcher = pattern.matcher(mydata);
if (matcher.find()) {
return matcher.group(1);
}
return null;
}
}
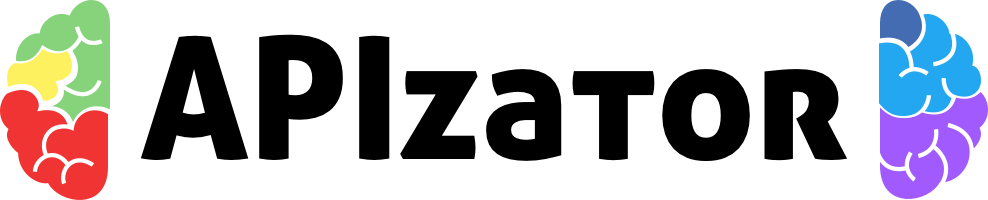
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* How to extract a substring using regex
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/4662265">https://stackoverflow.com/a/4662265</a>
*/
public class APIzator4662265 {
public static void extractSubstring(String mydata) throws Exception {
Pattern pattern = Pattern.compile("'(.*?)'");
Matcher matcher = pattern.matcher(mydata);
if (matcher.find()) {
System.out.println(matcher.group(1));
}
}
}