[Q#47210189][A#47210710] How to loop the following code?
https://stackoverflow.com/q/47210189
I'm really new to java and I cannot find a way around this. I want to make a program that tells you that a number is either positive or negative, regardless if it is int or double. But after the program is executed, I want it to loop and ask again for input from the user, to execute the code again and again and again, as long as there is user input. Can I do that in java?
Answer
https://stackoverflow.com/a/47210710
Here is a one of the approach which is good start for you to understand what wonders pattern matching can do in Java and it can be improved by testing it against exhaustive data points. This also shows how to use while-loop, overloading methods and ternary operator instead of nested if-then-else. As you are learning, you should also use debugging feature of editors and also use system.out.println to understand what code is doing. I am ending the program when user presses just enter (empty string). Sample Run:
APIzation

import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
String userInput = "Input your number: ";
System.out.print(userInput);
String input = scanner.nextLine();
// look for integer (+ve, -ve or 0)
if (input.matches("^-?[0-9]+$")) {
int z = Integer.parseInt(input);
System.out.println(display(z));
// look for double (+ve, -ve or 0)
} else if (input.matches("^-?([0-9]+\\.[0-9]+|[0-9]+)$")) {
double z = Double.parseDouble(input);
System.out.println(display(z));
// look for end of program by user
} else if (input.equals("")) {
System.out.println("Good Bye!!");
break;
// look for bad input
} else {
System.out.println("Hey! Only numbers!");
}
}
scanner.close();
}
// handle integer and display message appropriately
private static String display(int d) {
return (d>0) ? (d + " is positive") : (d<0) ? (d + " is negative") : (d + " is equal to 0");
}
// handle double and display message appropriately
private static String display(double d) {
return (d>0) ? (d + " is positive") : (d<0) ? (d + " is negative") : (d + " is equal to 0");
}
}
Input your number: 0
0 is equal to 0
Input your number: 0.0
0.0 is equal to 0
Input your number: -0
0 is equal to 0
Input your number: -0.0
-0.0 is equal to 0
Input your number: 12
12 is positive
Input your number: -12
-12 is negative
Input your number: 12.0
12.0 is positive
Input your number: -12.0
-12.0 is negative
Input your number: 12-12
Hey! Only numbers!
Input your number: ---12
Hey! Only numbers!
Input your number:
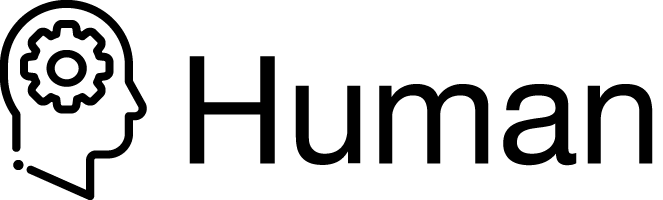
package com.stackoverflow.api;
import java.util.Scanner;
public class Human47210710 {
public static void loopCode() {
Scanner scanner = new Scanner(System.in);
while (true) {
String userInput = "Input your number: ";
System.out.print(userInput);
String input = scanner.nextLine();
// look for integer (+ve, -ve or 0)
if (input.matches("^-?[0-9]+$")) {
int z = Integer.parseInt(input);
System.out.println(display(z));
// look for double (+ve, -ve or 0)
} else if (input.matches("^-?([0-9]+\\.[0-9]+|[0-9]+)$")) {
double z = Double.parseDouble(input);
System.out.println(display(z));
// look for end of program by user
} else if (input.equals("")) {
System.out.println("Good Bye!!");
break;
// look for bad input
} else {
System.out.println("Hey! Only numbers!");
}
}
scanner.close();
}
// handle integer and display message appropriately
private static String display(int d) {
return (d > 0)
? (d + " is positive")
: (d < 0) ? (d + " is negative") : (d + " is equal to 0");
}
// handle double and display message appropriately
private static String display(double d) {
return (d > 0)
? (d + " is positive")
: (d < 0) ? (d + " is negative") : (d + " is equal to 0");
}
}
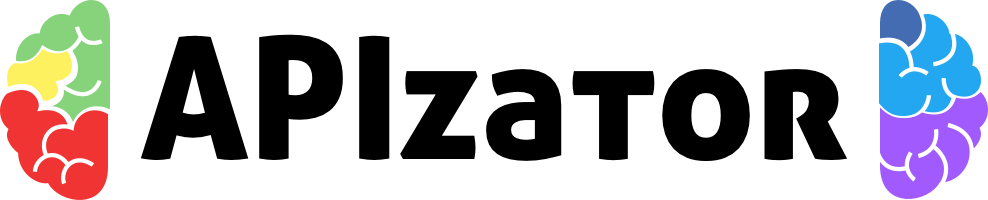
package com.stackoverflow.api;
import java.util.Scanner;
/**
* How to loop the following code?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/47210710">https://stackoverflow.com/a/47210710</a>
*/
public class APIzator47210710 {
public static void loopCode(String userInput) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print(userInput);
String input = scanner.nextLine();
// look for integer (+ve, -ve or 0)
if (input.matches("^-?[0-9]+$")) {
int z = Integer.parseInt(input);
System.out.println(display(z));
// look for double (+ve, -ve or 0)
} else if (input.matches("^-?([0-9]+\\.[0-9]+|[0-9]+)$")) {
double z = Double.parseDouble(input);
System.out.println(display(z));
// look for end of program by user
} else if (input.equals("")) {
System.out.println("Good Bye!!");
break;
// look for bad input
} else {
System.out.println("Hey! Only numbers!");
}
}
scanner.close();
}
// handle integer and display message appropriately
private static String display(int d) {
return (d > 0)
? (d + " is positive")
: (d < 0) ? (d + " is negative") : (d + " is equal to 0");
}
// handle double and display message appropriately
private static String display(double d) {
return (d > 0)
? (d + " is positive")
: (d < 0) ? (d + " is negative") : (d + " is equal to 0");
}
}