[Q#5652344][A#5652385] How can I use a custom font in Java?
https://stackoverflow.com/q/5652344
I wrote a program in Java that uses a special font that by default doesn't exist on any operating system. Is it possible in Java to add this special font to the operation system? For example, in Windows, to copy this font to the special Fonts folder. If it is possible, how?
Answer
https://stackoverflow.com/a/5652385
If you include a font file (otf, ttf, etc.) in your package, you can use the font in your application via the method described here: Oracle Java SE 6: java.awt.Font There is a tutorial available from Oracle that shows this example: I would probably wrap this up in some sort of resource loader though as to not reload the file from the package every time you want to use it. An answer more closely related to your original question would be to install the font as part of your application's installation process. That process will depend on the installation method you choose. If it's not a desktop app you'll have to look into the links provided.
APIzation

try {
GraphicsEnvironment ge =
GraphicsEnvironment.getLocalGraphicsEnvironment();
ge.registerFont(Font.createFont(Font.TRUETYPE_FONT, new File("A.ttf")));
} catch (IOException|FontFormatException e) {
//Handle exception
}
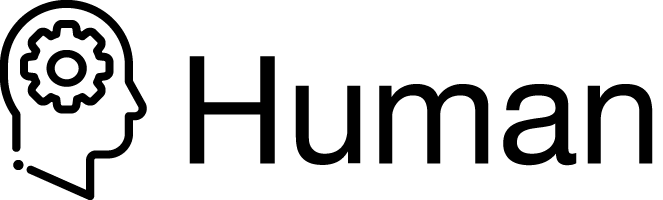
package com.stackoverflow.api;
import java.awt.*;
import java.io.File;
import java.io.IOException;
public class Human5652385 {
public static void registerFont(String str1) {
try {
GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment();
ge.registerFont(Font.createFont(Font.TRUETYPE_FONT, new File(str1)));
} catch (IOException | FontFormatException e) {
//Handle exception
}
}
}
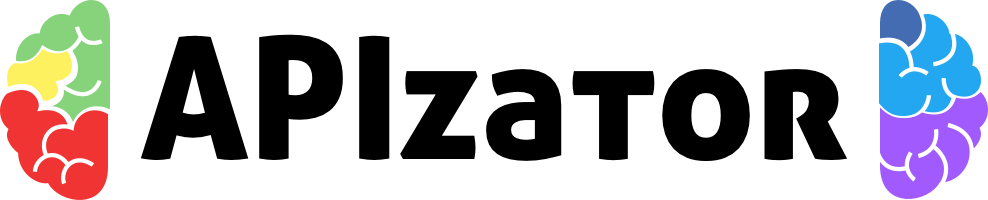
package com.stackoverflow.api;
import java.awt.Font;
import java.awt.FontFormatException;
import java.awt.GraphicsEnvironment;
import java.io.File;
import java.io.IOException;
/**
* How can I use a custom font in Java?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/5652385">https://stackoverflow.com/a/5652385</a>
*/
public class APIzator5652385 {
public static void useFont(String str1) throws Exception {
try {
GraphicsEnvironment ge = GraphicsEnvironment.getLocalGraphicsEnvironment();
ge.registerFont(Font.createFont(Font.TRUETYPE_FONT, new File(str1)));
} catch (IOException | FontFormatException e) {
// Handle exception
}
}
}