[Q#6341367][A#6341414] How to check if the string is a regular expression or not
https://stackoverflow.com/q/6341367
I have a string. How I can check if the string is a regular expression or contains regular expression or it is a normal string?
Answer
https://stackoverflow.com/a/6341414
The only reliable check you could do is if the String is a syntactically correct regular expression: Note, however, that this will result in true even for strings like Hello World and I'm not a regex, because technically they are valid regular expressions. The only cases where this will return false are strings that are not valid regular expressions, such as [unclosed character class or (unclosed group or +.
APIzation

boolean isRegex;
try {
Pattern.compile(input);
isRegex = true;
} catch (PatternSyntaxException e) {
isRegex = false;
}
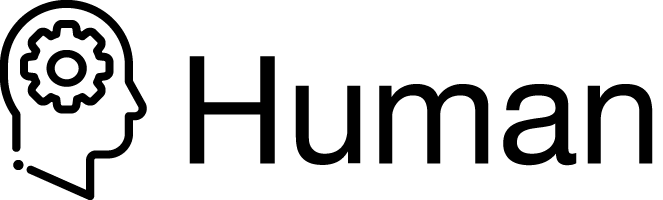
package com.stackoverflow.api;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
public class Human6341414 {
public static boolean isStringRegex(String input) {
//https://stackoverflow.com/a/6341414
boolean isRegex;
try {
Pattern.compile(input);
isRegex = true;
return isRegex;
} catch (PatternSyntaxException e) {
isRegex = false;
return isRegex;
}
}
}
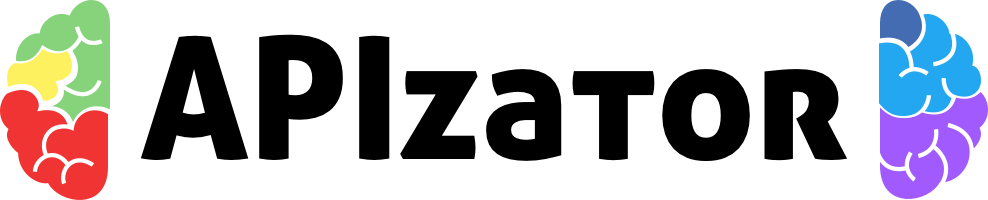
package com.stackoverflow.api;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
/**
* How to check if the string is a regular expression or not
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/6341414">https://stackoverflow.com/a/6341414</a>
*/
public class APIzator6341414 {
public static void check(String input) throws Exception {
boolean isRegex;
try {
Pattern.compile(input);
isRegex = true;
} catch (PatternSyntaxException e) {
isRegex = false;
}
}
}