[Q#6638321][A#6638330] How to exit two nested loops
https://stackoverflow.com/q/6638321
I have been using java for quite some time, yet my education in loops is somewhat lacking. I know how to create every loop that exists in java and break out of the loops as well. However, I've recently thought about this: Say I have two nested loops. Could I break out of both loops using just one break statement? Here is what I have so far. Is there any way to achieve this?
Answer
https://stackoverflow.com/a/6638330
In java you can use a label to specify which loop to break/continue:
APIzation

mainLoop:
while (goal <= 100) {
for (int i = 0; i < goal; i++) {
if (points > 50) {
break mainLoop;
}
points += i;
}
}
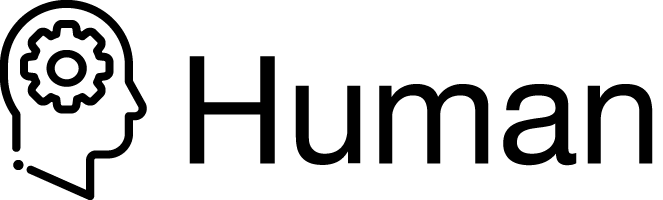
package com.stackoverflow.api;
public class Human6638330 {
public static void breakMainLoop(int pPoints, int goal) {
mainLoop:while (goal <= 100) {
for (int i = 0; i < goal; i++) {
if (pPoints > 50) {
break mainLoop;
}
pPoints += i;
}
}
}
}
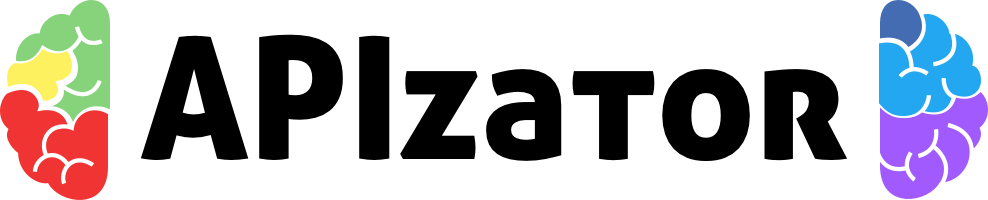
package com.stackoverflow.api;
/**
* How to exit two nested loops
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/6638330">https://stackoverflow.com/a/6638330</a>
*/
public class APIzator6638330 {
public static void exitLoop(int goal) throws Exception {
int points = 0;
mainLoop:while (goal <= 100) {
for (int i = 0; i < goal; i++) {
if (points > 50) {
break mainLoop;
}
points += i;
}
}
}
}