[Q#8963413][A#8963444] How to run Windows commands in JAVA and return the result text as a string
https://stackoverflow.com/q/8963413
Possible Duplicate: Get output from a process Executing DOS commands from Java I am trying to run a cmd command from within a JAVA console program e.g.: and then return the output of the command into a string in JAVA e.g. output:
Answer
https://stackoverflow.com/a/8963444
You can use the following code for this
APIzation

import java.io.*;
public class doscmd
{
public static void main(String args[])
{
try
{
Process p=Runtime.getRuntime().exec("cmd /c dir");
p.waitFor();
BufferedReader reader=new BufferedReader(
new InputStreamReader(p.getInputStream())
);
String line;
while((line = reader.readLine()) != null)
{
System.out.println(line);
}
}
catch(IOException e1) {e1.printStackTrace();}
catch(InterruptedException e2) {e2.printStackTrace();}
System.out.println("Done");
}
}
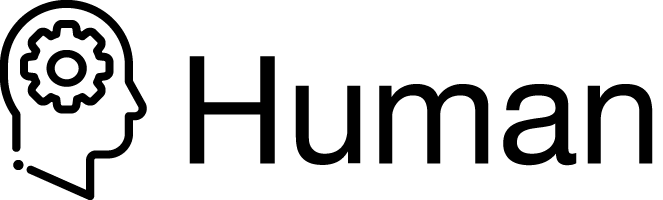
package com.stackoverflow.api;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Human8963444 {
public static String runCommand(String command)
throws IOException, InterruptedException {
Process p = Runtime.getRuntime().exec(command);
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(p.getInputStream())
);
String line;
StringBuilder sb = new StringBuilder();
while ((line = reader.readLine()) != null) {
sb.append(line);
}
return sb.toString();
}
}
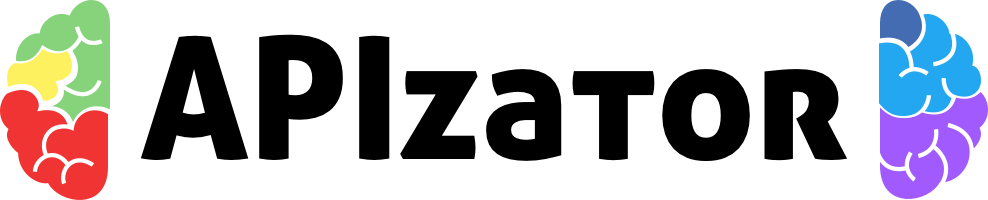
package com.stackoverflow.api;
import java.io.*;
/**
* How to run Windows commands in JAVA and return the result text as a string
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/8963444">https://stackoverflow.com/a/8963444</a>
*/
public class APIzator8963444 {
public static void runCommand() {
try {
Process p = Runtime.getRuntime().exec("cmd /c dir");
p.waitFor();
BufferedReader reader = new BufferedReader(
new InputStreamReader(p.getInputStream())
);
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e1) {
e1.printStackTrace();
} catch (InterruptedException e2) {
e2.printStackTrace();
}
System.out.println("Done");
}
}