[Q#9464323][A#9464552] how to extract a phone number for a string using regular expression?
https://stackoverflow.com/q/9464323
If I have a string that contains the following: This is 1 test 123-456-7890 I need to extract 1234567890 as a phone number. I don't want to extract the number 1 that is before test. How can I do that using regular expressions in java? I know a way but I am not sure if it is the best solution:
Answer
https://stackoverflow.com/a/9464552
The following code will check for the phone number in the string you mention and print it: But, as pointed out in other answers, many phone numbers (especially not international ones) will not match the pattern.
APIzation

String str = "This is 1 test 123-456-7890";
Pattern pattern = Pattern.compile("\\d{3}-\\d{3}-\\d{4}");
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
System.out.println(matcher.group(0));
}
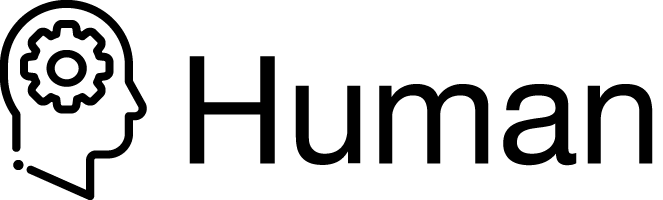
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Human9464552 {
public static String extractPhoneNumber(String str) {
Pattern pattern = Pattern.compile("\\d{3}-\\d{3}-\\d{4}");
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
return matcher.group(0);
}
return null;
}
}
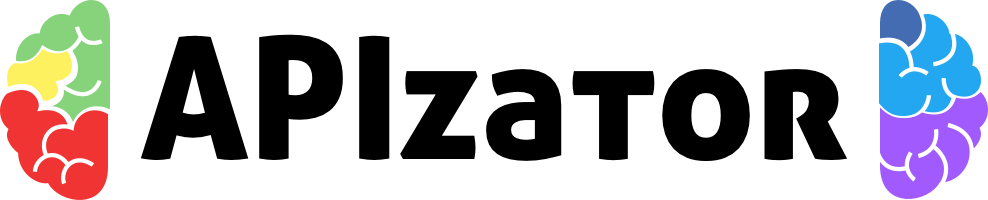
package com.stackoverflow.api;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* how to extract a phone number for a string using regular expression?
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/9464552">https://stackoverflow.com/a/9464552</a>
*/
public class APIzator9464552 {
public static void extractNumber(String str) throws Exception {
Pattern pattern = Pattern.compile("\\d{3}-\\d{3}-\\d{4}");
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
System.out.println(matcher.group(0));
}
}
}