[Q#9962420][A#9962492] How to get the 'place' of a Number in Java (eg. tens, thousands, etc)
https://stackoverflow.com/q/9962420
How can I determine the number places of a number and determine the number of a loop to run? For example, if i have an array int[] a= {123,342,122,333,9909} and int max = a.getMax() we get the value 9909. I want to get the number place value of 9909, which is the thousand-th place. For example… Here is my code, however it fails when it meets a zero between an integer…
Answer
https://stackoverflow.com/a/9962492
You can use logarithms.
APIzation

double[] values = {4, 77, 234, 4563, 13467, 635789};
for(int i = 0; i < values.length; i++)
{
double tenthPower = Math.floor(Math.log10(values[i]));
double place = Math.pow(10, tenthPower);
System.out.println(place);
}
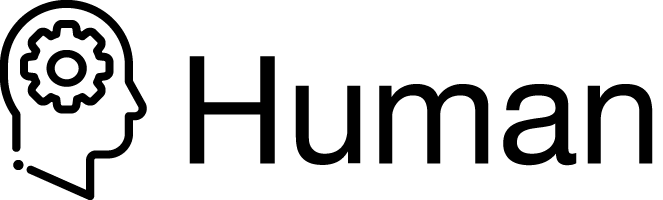
package com.stackoverflow.api;
public class Human9962492 {
public static void printPlaces(double[] values) {
for (int i = 0; i < values.length; i++) {
double tenthPower = Math.floor(Math.log10(values[i]));
double place = Math.pow(10, tenthPower);
System.out.println(place);
}
}
}
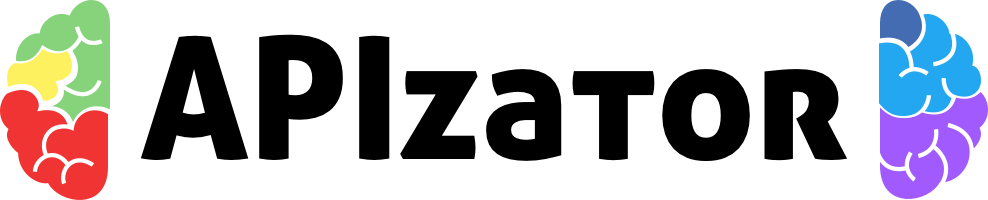
package com.stackoverflow.api;
/**
* How to get the 'place' of a Number in Java (eg. tens, thousands, etc)
*
* @author APIzator
* @see <a href="https://stackoverflow.com/a/9962492">https://stackoverflow.com/a/9962492</a>
*/
public class APIzator9962492 {
public static void getPlace(double[] values) throws Exception {
for (int i = 0; i < values.length; i++) {
double tenthPower = Math.floor(Math.log10(values[i]));
double place = Math.pow(10, tenthPower);
System.out.println(place);
}
}
}